REST API
The test bed’s REST API allows you to carry out most operations without connecting to its user interface. Its typical use case is to allow integration with automated processes enabling use cases such as automated testing and continuous integration. For test developers certain API operations may also be interesting as it allows them to more easily validate changes while developing test cases.
As a general note, identifying the operations’ caller and referring to existing data in the test bed is done via API keys. For operations that would involve choices and confirmations if done via their corresponding user interface screens, these are provided in each case as inputs of the relevant REST calls.
The REST API foresees the following types of operations:
Management of communities, organisations and systems (see Community management).
Management of configuration property definitions and values (see Configuration management).
Management of domains, specification groups, specifications and actors (see Domain management).
Management of conformance statements (see Conformance statement management).
Launch and management of test sessions (see Test session management).
Management of test suites (see Test suite management).
These sets of operations are documented in the sections that follow.
Note
Using the test bed’s REST API is an advanced feature that needs to first be enabled by your administrator to be available to you. If setting up your own test bed instance (for production or development) you may enable this by setting the AUTOMATION_API_ENABLED property to true or through the user interface after switching to the test bed administrator account.
Community management
The community management operations allow you to manage the test bed’s communities, organisations and systems. They are useful if you want to have processes external to the test bed manage such information without needing manual actions through the test bed’s user interface. Specifically you can:
All community management operations use API keys to authorise calls and determine the information to be updated. Two types of API keys are used, depending on the operation:
The key identifying a community, for all operations that a community administrator can do through the user interface.
The master API key, for selected top-level operations that are normally reserved for the overall test bed administrator.
The sections that follow provide instructions and examples for each operation.
createCommunity
The createCommunity operation is used to create a new community. To use it make an HTTP PUT
to path /api/rest/community
and include in your request an HTTP header named ITB_API_KEY
set to your master API key.
In the request’s body you specify the information of the new community, of which the shortName
and fullName
are mandatory. Other information you
would typically be providing, although not mandatory, would be the community’s description
and the domain
it relates to, the latter identified through
the domain’s API key. For the full set of information you can manage check the payload’s schema.
The following example shows how you can create a community with the provided data:
{
"shortName": "EUPO community",
"fullName": "European Purchase Order community",
"description": "Community for the conformance testing of the European Purchase Order specification.",
"supportEmail": "support@eupo.eu",
"interactionNotifications": true,
"domain": "3F471BA2XDD33X4FCEX8045X1D8039E6B92A"
}
Calling this operation, the test bed will create the community and link it with the identified domain. Once complete, the operation will respond with the community’s assigned API key:
{
"apiKey": "B277E210X2FB4X4BD7X88B6X951504F45F8F"
}
It is possible to avoid the automatic API key assignment by defining an API key to use as part of the input. This could be useful if you are configuring a test bed instance based on fixed data and you want to ensure assigned API keys have specific, known values.
{
"shortName": "EUPO community",
"fullName": "European Purchase Order community",
"description": "Community for the conformance testing of the European Purchase Order specification.",
"supportEmail": "support@eupo.eu",
"interactionNotifications": true,
"domain": "3F471BA2XDD33X4FCEX8045X1D8039E6B92A",
"apiKey": "A_FIXED_API_KEY_FOR_THE_COMMUNITY"
}
In this case the test bed will use the provided API key unless it is found to already exist, in which case a new one will be generated instead. Regardless, the operation will still report the API key that was assigned.
createCommunity - request schema
The payload of the createCommunity operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/createCommunity_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the createCommunity operation request payload",
"type": "object",
"properties": {
"shortName": {
"description": "The community's short name.",
"type": "string"
},
"fullName": {
"description": "The community's full name.",
"type": "string"
},
"description": {
"description": "The community's description.",
"type": "string"
},
"supportEmail": {
"description": "A support email address to receive community-specific notifications at.",
"type": "string"
},
"interactionNotifications": {
"description": "Whether to receive notifications for pending interactions by email at the support mailbox (see supportEmail).",
"type": "boolean",
"default": false
},
"apiKey": {
"description": "The API key to set for the community. If this key is already assigned, a new one will be generated.",
"type": "string"
},
"domain": {
"description": "The API key identifying a domain that should be linked to the community.",
"type": "string"
}
},
"required": [
"shortName",
"fullName"
],
"additionalProperties": false
}
createCommunity - response schema
The payload of the createCommunity operation’s response is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/apiKey_response",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema to signal an assigned API key response",
"type": "object",
"properties": {
"apiKey": {
"description": "The API key value.",
"type": "string"
}
},
"required": [
"apiKey"
],
"additionalProperties": false
}
updateCommunity
The updateCommunity operation is used to update an existing community. It exists in two variants which differ in how the target community is identified, the updates that you can do, and the API key you will use for authorisation.
The first variant assumes that you are performing an update as a community administrator would. In this case you can only update your own community,
and cannot change the community’s assigned domain. You call the operation by making an HTTP POST
to path /api/rest/community
and setting an
HTTP header named ITB_API_KEY
to your community API key.
The second variant allows you to perform tasks reserved for the test bed administrator. This means that you can select any existing community in the
test bed, and also change or remove its assigned domain. In this case you call the operation by making an HTTP POST
to path
/api/rest/community/{community}
, setting {community}
to the target community’s API key. The ITB_API_KEY
HTTP header needs to be set
with the master API key.
When calling this operation, regardless of the specific variant, all input properties are optional. You specify the properties that you want to update, and for the ones that are to be left unchanged you don’t include them. In case you want to remove a value, for example unlinking the community from its current domain, you would specify the property in question as an empty string.
The following example illustrates an update of the community’s supportEmail
, leaving all other information unchanged:
{
"supportEmail": "support-mailbox@eupo.eu"
}
In case you want to altogether remove the support mailbox, you specify it’s value as an empty string:
{
"supportEmail": ""
}
The full set of properties that can be updated through this operation are detailed in the payload’s schema.
The operation’s response is empty, signalling through a status 200
(OK) that the update was successfully made.
updateCommunity - request schema
The payload of the updateCommunity operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/updateCommunity_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the updateCommunity operation request payload",
"properties": {
"shortName": {
"description": "The community's short name. Skip this if no update should be made.",
"type": "string"
},
"fullName": {
"description": "The community's full name. Skip this if no update should be made.",
"type": "string"
},
"description": {
"description": "The community's description. Skip this if no update should be made or set to an empty string to remove the current value.",
"type": "string"
},
"supportEmail": {
"description": "A support email address to receive community-specific notifications at. Skip this if no update should be made or set to an empty string to remove the current value.",
"type": "string"
},
"interactionNotifications": {
"description": "Whether to receive notifications for pending interactions by email at the support mailbox (see supportEmail). Skip this if no update should be made.",
"type": "boolean"
},
"domain": {
"description": "The API key identifying a domain that should be linked to the community. Skip this if no update should be made or set to an empty string to unlink the community from its existing domain (without deleting the domain).",
"type": "string"
}
}
}
deleteCommunity
The deleteCommunity operation is used to delete an existing community. To use it make an HTTP DELETE
to path /api/rest/community/{community}
,
setting {community}
to the target community’s API key. In addition, you must include in your request an HTTP header named
ITB_API_KEY
set to your master API key.
This operation takes no payload when making a request. If the target community has been successfully deleted, it will respond with an
empty body and a status of 200
(OK) to signal that the deletion was successful.
createOrganisation
The createOrganisation operation is used to create a new organisation. To use it make an HTTP PUT
to path /api/rest/organisation
and include in your request an HTTP header named ITB_API_KEY
set to your community API key. This API key identifies the community
within which the organisation will be created.
In the request’s body you specify the information of the new organisation, of which the shortName
and fullName
are mandatory as defined
in the payload’s schema.
The following example shows how you can create an organisation with the provided data:
{
"shortName": "ACME",
"fullName": "ACME Ltd."
}
Calling this operation, the test bed will create the organisation and respond with its assigned API key:
{
"apiKey": "B277E210X2FB4X4BD7X88B6X951504F45F8F"
}
It is possible to avoid the automatic API key assignment by defining an API key to use as part of the input. This could be useful if you are configuring a test bed instance based on fixed data and you want to ensure assigned API keys have specific, known values.
{
"shortName": "ACME",
"fullName": "ACME Ltd.",
"apiKey": "A_FIXED_API_KEY_FOR_THE_ORGANISATION"
}
In this case the test bed will use the provided API key unless it is found to already exist, in which case a new one will be generated instead. Regardless, the operation will still report the API key that was assigned.
createOrganisation - request schema
The payload of the createOrganisation operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/createOrganisation_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the createOrganisation operation request payload",
"type": "object",
"properties": {
"shortName": {
"description": "The organisation's short name.",
"type": "string"
},
"fullName": {
"description": "The organisation's full name.",
"type": "string"
},
"apiKey": {
"description": "The API key to set for the organisation. If this key is already assigned, a new one will be generated.",
"type": "string"
}
},
"required": [
"shortName",
"fullName"
],
"additionalProperties": false
}
createOrganisation - response schema
The payload of the createOrganisation operation’s response is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/apiKey_response",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema to signal an assigned API key response",
"type": "object",
"properties": {
"apiKey": {
"description": "The API key value.",
"type": "string"
}
},
"required": [
"apiKey"
],
"additionalProperties": false
}
updateOrganisation
The updateOrganisation operation is used to update an existing organisation. To use it make an HTTP POST
to path /api/rest/organisation/{organisation}
,
setting {organisation}
to the target organisation’s API key. You also need to include in your request an HTTP header named ITB_API_KEY
set to the
community API key of the community containing the organisation.
When calling this operation all input properties are optional. You specify the properties that you want to update, and for the ones that are to be left unchanged you don’t include them.
The following example illustrates an update of the organisation’s fullName
, leaving other information unchanged:
{
"fullName": "ACME Limited"
}
The properties that can be updated through this operation are detailed in the payload’s schema.
The operation’s response is empty, signalling through a status 200
(OK) that the update was successfully made.
updateOrganisation - request schema
The payload of the updateOrganisation operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/updateOrganisation_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the updateOrganisation operation request payload",
"properties": {
"shortName": {
"description": "The organisation's short name. Skip this if no update should be made.",
"type": "string"
},
"fullName": {
"description": "The organisation's full name. Skip this if no update should be made.",
"type": "string"
}
},
"additionalProperties": false
}
deleteOrganisation
The deleteOrganisation operation is used to delete an existing organisation. To use it make an HTTP DELETE
to path /api/rest/organisation/{organisation}
,
setting {organisation}
to the target organisation’s API key. In addition, you must include in your request an HTTP header named
ITB_API_KEY
set to your community API key.
This operation takes no payload when making a request. If the target organisation has been successfully deleted, it will respond with an
empty body and a status of 200
(OK) to signal that the deletion was successful.
createSystem
The createSystem operation is used to create a new system. To use it make an HTTP PUT
to path /api/rest/system
and include in your request an HTTP header named ITB_API_KEY
set to your community API key. This API key identifies the community
within which the system will be created.
In the request’s body you specify the information of the new system, of which the shortName
and fullName
are mandatory as defined
in the payload’s schema. In addition, you must include the organisation
within which
the system will be created, setting its value to the organisation’s API key.
The following example shows how you can create a system under a given organisation with the provided data:
{
"shortName": "PS",
"fullName": "Purchasing system",
"description": "ACME's flagship purchasing solution.",
"version": "v1.0",
"organisation": "B277E210X2FB4X4BD7X88B6X951504F45F8F"
}
Calling this operation, the test bed will create the system and respond with its assigned API key:
{
"apiKey": "3F471BA2XDD33X4FCEX8045X1D8039E6B92A"
}
It is possible to avoid the automatic API key assignment by defining an API key to use as part of the input. This could be useful if you are configuring a test bed instance based on fixed data and you want to ensure assigned API keys have specific, known values.
{
"shortName": "PS",
"fullName": "Purchasing system",
"apiKey": "A_FIXED_API_KEY_FOR_THE_SYSTEM"
}
In this case the test bed will use the provided API key unless it is found to already exist, in which case a new one will be generated instead. Regardless, the operation will still report the API key that was assigned.
createSystem - request schema
The payload of the createSystem operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/createSystem_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the createSystem operation request payload",
"type": "object",
"properties": {
"shortName": {
"description": "The system's short name.",
"type": "string"
},
"fullName": {
"description": "The system's full name.",
"type": "string"
},
"description": {
"description": "The system's description.",
"type": "string"
},
"version": {
"description": "The system's version information.",
"type": "string"
},
"apiKey": {
"description": "The API key to set for the system. If this key is already assigned, a new one will be generated.",
"type": "string"
},
"organisation": {
"description": "The API key identifying the organisation in which to create the system.",
"type": "string"
}
},
"required": [
"shortName",
"fullName",
"organisation"
],
"additionalProperties": false
}
createSystem - response schema
The payload of the createSystem operation’s response is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/apiKey_response",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema to signal an assigned API key response",
"type": "object",
"properties": {
"apiKey": {
"description": "The API key value.",
"type": "string"
}
},
"required": [
"apiKey"
],
"additionalProperties": false
}
updateSystem
The updateSystem operation is used to update an existing system. To use it make an HTTP POST
to path /api/rest/system/{system}
,
setting {system}
to the target system’s API key. You also need to include in your request an HTTP header named ITB_API_KEY
set to the
community API key of the community containing the system.
When calling this operation all input properties are optional. You specify the properties that you want to update, and for the ones that are to be left unchanged you don’t include them. In case you want to remove a value you would specify the property in question as an empty string.
The following example illustrates an update of the system’s fullName
, leaving other information unchanged:
{
"fullName": "Amazing purchasing system"
}
In case you want to altogether remove a property, such as the the system’s description, you specify it’s value as an empty string:
{
"description": ""
}
The properties that can be updated through this operation are detailed in the payload’s schema.
The operation’s response is empty, signalling through a status 200
(OK) that the update was successfully made.
updateSystem - request schema
The payload of the updateSystem operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/updateSystem_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the updateSystem operation request payload",
"properties": {
"shortName": {
"description": "The system's short name. Skip this if no update should be made.",
"type": "string"
},
"fullName": {
"description": "The system's full name. Skip this if no update should be made.",
"type": "string"
},
"description": {
"description": "The system's description. Skip this if no update should be made or provide as an empty string to remove the current value.",
"type": "string"
},
"version": {
"description": "The system's version information. Skip this if no update should be made or provide as an empty string to remove the current value.",
"type": "string"
}
},
"additionalProperties": false
}
deleteSystem
The deleteSystem operation is used to delete an existing system. To use it make an HTTP DELETE
to path /api/rest/system/{system}
,
setting {system}
to the target system’s API key. In addition, you must include in your request an HTTP header named
ITB_API_KEY
set to your community API key.
This operation takes no payload when making a request. If the target system has been successfully deleted, it will respond with an
empty body and a status of 200
(OK) to signal that the deletion was successful.
Configuration management
The test bed provides the possibility to manage configuration entries via its REST API. Two types of configuration actions are available:
Managing the definition of configuration properties.
Setting the values of configuration properties.
In terms of defining configuration properties you can:
Once properties are defined you can manage their values for specific organisations and systems through the configure operation.
configure
The configure operation allows you to adapt the values for configuration entries at all levels, specifically:
At domain level, for configuration applying to the overall test configuration.
At organisation level, for configuration pertinent to a specific organisation.
At system level, for configuration pertinent to a specific organisation’s system.
At statement level, for configuration relevant to a conformance statement linking a system and specification actor.
For properties at these levels this operation allows you to create, update and delete values. The exception is the creation and deletion of domain properties, for which you need to use the dedicated createDomainProperty and deleteDomainProperty operations.
To call the configure operation make an HTTP POST
to path /api/rest/configure
. To authorise the operation and identify the properties to manage,
you must include in your request an HTTP header named ITB_API_KEY
set to your community API key.
In the request’s payload you can provide any of the following properties depending on what configuration you want to update:
domainProperties
as an array ofkey
andvalue
pairs. In case the domain of the property cannot be determined you can also setdomain
with the relevant domain’s API key.organisationProperties
as an array where each item defines the relevantorganisation
API key followed by an array ofkey
andvalue
pairs.systemProperties
as an array where each item defines the relevantsystem
API key followed by an array ofkey
andvalue
pairs.statementProperties
as an array where each item defines the relevantsystem
andactor
API keys followed by an array ofkey
andvalue
pairs.
The above properties are optional, allowing you to specify precisely what you want to update. Regarding the key
and value
pairs, the key
always
refers to the unique identifier of the property in question (the same as what is used to refer to it in test cases), for which the provided value
will be
applied. The specific action to take place is determined as follows:
If
value
is provided, the property will be updated or created if not existing.If
value
is not provided and an existing value is defined it will be deleted.
Recall that in the case of domain properties, only updates of existing properties are allowed to take place through this operation. In addition, only properties that are simple text values can be managed via the API.
The following sample is a JSON request to update a property at each configuration level.
{
"domainProperties": [
{ "key": "sampleProperty", "value": "newValue" }
],
"organisationProperties": [
{
"organisation": "ORGANISATION_API_KEY",
"properties": [
{ "key": "sampleProperty", "value": "newValue" }
]
}
],
"systemProperties": [
{
"system": "SYSTEM_API_KEY",
"properties": [
{ "key": "sampleProperty", "value": "newValue" }
]
}
],
"statementProperties": [
{
"system": "SYSTEM_API_KEY",
"actor": "ACTOR_API_KEY",
"properties": [
{ "key": "sampleProperty", "value": "newValue" }
]
}
]
}
As mentioned previously you can also focus on specific properties and update multiple in one go. The following sample updates two organisation properties for two different organisations.
{
"organisationProperties": [
{
"organisation": "ORGANISATION_API_KEY_1",
"properties": [
{ "key": "sampleProperty1", "value": "newValue1" },
{ "key": "sampleProperty2", "value": "newValue2" }
]
},
{
"organisation": "ORGANISATION_API_KEY_2",
"properties": [
{ "key": "sampleProperty1", "value": "newValue1" },
{ "key": "sampleProperty2", "value": "newValue2" }
]
}
]
}
To delete properties, simply identify the properties in question and omit the value
.
{
"organisationProperties": [
{
"organisation": "ORGANISATION_API_KEY",
"properties": [
{ "key": "sampleProperty1" },
{ "key": "sampleProperty2" }
]
}
]
}
Once the configure operation has completed you will receive a JSON response providing feedback on the result. If all actions could be carried out the payload
will be en empty JSON object. In case warnings were encountered (for example an organisation not being found, or a target property not being a simple text value),
these will be reported in a string array named messages
.
{
"messages": [
"No conformance statement defined for system [SYSTEM_API_KEY] and actor [ACTOR_API_KEY]."
]
}
configure - request schema
The payload of the configure operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/configure_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the configure operation request payload",
"type": "object",
"properties": {
"domainProperties": {
"description": "Updates to domain properties.",
"type": "array",
"items": {
"$ref": "#/definitions/domainConfigurationEntry"
}
},
"organisationProperties": {
"description": "Updates to organisation properties.",
"type": "array",
"items": {
"$ref": "#/definitions/organisationConfigurationEntry"
}
},
"systemProperties": {
"description": "Updates to system properties.",
"type": "array",
"items": {
"$ref": "#/definitions/systemConfigurationEntry"
}
},
"statementProperties": {
"description": "Updates to conformance statement properties.",
"type": "array",
"items": {
"$ref": "#/definitions/conformanceStatementConfigurationEntry"
}
}
},
"additionalProperties": false,
"definitions": {
"configurationEntry": {
"type": "object",
"properties": {
"key": { "type": "string" },
"value": { "type": "string" }
},
"required": [ "key" ],
"additionalProperties": false
},
"domainConfigurationEntry": {
"description": "Configuration update to apply to a given domain property.",
"type": "object",
"properties": {
"key": {
"description": "The unique key used to identify the configuration property.",
"type": "string"
},
"value": {
"description": "The value to apply for the configuration property (if missing this signals a delete of the existing value).",
"type": "string"
},
"domain": {
"description": "The API key to identify the domain under which this property is defined (in case of multiple domains defining the same property).",
"type": "string"
}
},
"required": [
"key"
],
"additionalProperties": false
},
"organisationConfigurationEntry": {
"type": "object",
"properties": {
"organisation": { "type": "string" },
"properties": { "type": "array", "items": { "$ref": "#/definitions/configurationEntry" }, "minItems": 1 }
},
"required": [ "organisation", "properties" ],
"additionalProperties": false
},
"systemConfigurationEntry": {
"type": "object",
"properties": {
"system": { "type": "string" },
"properties": { "type": "array", "items": { "$ref": "#/definitions/configurationEntry" }, "minItems": 1 }
},
"required": [ "system", "properties" ],
"additionalProperties": false
},
"conformanceStatementConfigurationEntry": {
"type": "object",
"properties": {
"system": { "type": "string" },
"actor": { "type": "string" },
"properties": { "type": "array", "items": { "$ref": "#/definitions/configurationEntry" }, "minItems": 1 }
},
"required": [ "system", "actor", "properties" ],
"additionalProperties": false
}
}
}
configure - response schema
The payload of the configure operation’s response is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/configure_response",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the configure operation response payload",
"type": "object",
"properties": {
"messages": {
"description": "The list of warning messages for updates that could not be carried out.",
"type": "array",
"items": {
"type": "string"
}
}
},
"additionalProperties": false
}
createDomainProperty
The createDomainProperty operation is used to define a new domain property. To use it make an HTTP PUT
to path /api/rest/configure/domain
and include in your request an HTTP header named ITB_API_KEY
set to your community API key. This API key identifies a community
that has access to manage the domain in question, either by being directly linked to it or by being linked to no domain.
In the request’s body you specify the information of the domain property, of which the key
and value
are mandatory as defined
in the payload’s schema. In case the target domain cannot be determined by the community
linked to the provided ITB_API_KEY
, you also need to specify the domain
property, set with the API key of the target domain.
The following example shows how you can create a domain property with the provided data:
{
"key": "validationService",
"value": "http://test-services/services/validation?wsdl",
"description": "The WSDL address of the validation service used in test cases."
}
Calling this operation, the test bed will create the property and respond with a 200
(OK) response if successful.
createDomainProperty - request schema
The payload of the createDomainProperty operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/createDomainProperty_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the createDomainProperty operation request payload",
"type": "object",
"properties": {
"key": {
"description": "The unique key used to identify the parameter.",
"type": "string"
},
"value": {
"description": "The value to apply for the parameter.",
"type": "string"
},
"description": {
"description": "The parameter's description.",
"type": "string"
},
"inTests": {
"description": "Whether the parameter should be exposed to test sessions.",
"type": "boolean",
"default": true
},
"domain": {
"description": "The API key identifying the domain of the parameter. This is required if the community API key used for authentication refers to a community that is not linked to a specific domain.",
"type": "string"
}
},
"required": [
"key",
"value"
],
"additionalProperties": false
}
updateDomainProperty
The updateDomainProperty operation is used to update the definition of a domain property. To use it make an HTTP POST
to path /api/rest/configure/domain
and include in your request an HTTP header named ITB_API_KEY
set to your community API key. This API key identifies a community
that has access to manage the domain in question, either by being directly linked to it or by being linked to no domain.
Note
Updating the value of domain properties can also be achieved using the configure operation.
When calling this operation all input properties are optional except for the key
that is used to identify the target property. In addition, providing domain
and setting it with a domain API key might be needed, in case the relevant domain cannot be determined. For all other properties you specify the ones that you want to update,
and for those that are to be left unchanged you don’t include them. In case you want to remove a value you would specify the property in question as an empty string.
The following example illustrates an update of a domain property’s description
, leaving other information unchanged:
{
"key": "validationService",
"description": "The validation service address."
}
In case you want to altogether remove a value, you specify it as an empty string:
{
"key": "validationService",
"description": ""
}
The properties that can be updated through this operation are detailed in the payload’s schema.
The operation’s response is empty, signalling through a status 200
(OK) that the update was successfully made.
updateDomainProperty - request schema
The payload of the updateDomainProperty operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/updateDomainProperty_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the updateDomainProperty operation request payload",
"type": "object",
"properties": {
"key": {
"description": "The unique key used to identify the parameter.",
"type": "string"
},
"value": {
"description": "The new value to apply for the parameter. Skip this if no update should be made.",
"type": "string"
},
"description": {
"description": "The parameter's description. Skip this if no update should be made or set with an empty string to delete the current value.",
"type": "string"
},
"inTests": {
"description": "Whether the parameter should be exposed to test sessions. Skip this if no update should be made.",
"type": "boolean"
},
"domain": {
"description": "The API key identifying the domain of the parameter. This is required if the community API key used for authentication refers to a community that is not linked to a specific domain.",
"type": "string"
}
},
"required": [
"key"
],
"additionalProperties": false
}
deleteDomainProperty
The deleteDomainProperty operation is used to delete the definition of a domain property. To use it make an HTTP DELETE
to path /api/rest/configure/domain/{parameter}
,
setting {parameter}
to the target property’s key. In addition, you must include in your request an HTTP header named ITB_API_KEY
set to your community API key.
This API key identifies a community that has access to manage the domain in question, either by being directly linked to it or by being linked to no domain.
In case the provided community API key identifies a community without a linked domain, you also need to identify the target domain. This is done by adapting the operation’s path to
/api/rest/configure/domain/{domain}/{parameter}
where the additional {domain}
placeholder is set with the target domain’s API key.
This operation takes no payload when making a request. If the target property has been successfully deleted, it will respond with an
empty body and a status of 200
(OK) to signal that the deletion was successful.
createOrganisationProperty
The createOrganisationProperty operation is used to define a new organisation property for a community. To use it make an HTTP PUT
to path /api/rest/configure/organisation
and include in your request an HTTP header named ITB_API_KEY
set to your community API key.
In the request’s body you specify the information of the organisation property, of which the key
is mandatory. The full set of information you can set for the property is defined
in the payload’s schema.
The following example shows how you can create an organisation property with the provided data:
{
"key": "vatNumber",
"name": "VAT number",
"description": "The VAT number of the supplier.",
"required": true,
"editableByUsers": true,
"inTests": true
}
Other more advanced options include specifying the property’s allowed values, default value, and dependencies. In the latter case, dependsOn
is set with the key of the property
that the current one depends upon:
{
"key": "vatType",
"name": "VAT regime",
"description": "The VAT regime the suppler is subject to.",
"required": true,
"editableByUsers": true,
"inTests": true,
"allowedValues": [
{ "value": "full", "label": "Full regime" },
{ "value": "minimal", "label": "Minimal regime" },
{ "value": "none", "label": "Exempt" }
],
"defaultValue": "full",
"dependsOn": "considerTax",
"dependsOnValue": "Y"
}
Calling this operation, the test bed will create the property and respond with a 200
(OK) response if successful.
createOrganisationProperty - request schema
The payload of the createOrganisationProperty operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/organisationPropertyInfo_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the createOrganisationProperty and updateOrganisationProperty operation request payloads",
"type": "object",
"properties": {
"key": {
"description": "The unique key used to identify the property.",
"type": "string"
},
"name": {
"description": "The property's name. If not set this will be the same as the property's key.",
"type": "string"
},
"description": {
"description": "The property's description.",
"type": "string"
},
"required": {
"description": "Whether the property must be set before executing test sessions.",
"type": "boolean",
"default": true
},
"editableByUsers": {
"description": "Whether the property can be set by organisation users.",
"type": "boolean",
"default": true
},
"inTests": {
"description": "Whether the property should be exposed to test sessions.",
"type": "boolean",
"default": false
},
"inExports": {
"description": "Whether the property should be included in exports.",
"type": "boolean",
"default": false
},
"inSelfRegistration": {
"description": "Whether the property should be requested during self-registration.",
"type": "boolean",
"default": false
},
"hidden": {
"description": "Whether the property should be hidden from organisation users.",
"type": "boolean",
"default": false
},
"allowedValues": {
"description": "The list of allowed values for the property.",
"type": "array",
"items": {
"$ref": "#/definitions/allowedPropertyValue"
}
},
"displayOrder": {
"description": "The display order of the property when presented to users.",
"type": "number"
},
"dependsOn": {
"description": "The key of another property upon which this property's applicability depends on (see also dependsOnValue).",
"type": "string"
},
"dependsOnValue": {
"description": "If the dependsOn property is set, this is the value that the referred-to property needs to have for this property to be considered applicable.",
"type": "string"
},
"defaultValue": {
"description": "The default value of the property when a new organisation is created.",
"type": "string"
}
},
"required": [
"key"
],
"additionalProperties": false,
"definitions": {
"allowedPropertyValue": {
"description": "An entry for a configuration property representing an allowed value and its display label.",
"type": "object",
"properties": {
"value": {
"description": "The property value.",
"type": "string"
},
"label": {
"description": "The property's display label.",
"type": "string"
}
},
"required": [
"value",
"label"
],
"additionalProperties": false
}
}
}
updateOrganisationProperty
The updateOrganisationProperty operation is used to update the definition of an organisation property. To use it make an HTTP POST
to path /api/rest/configure/organisation
and include in your request an HTTP header named ITB_API_KEY
set to your community API key.
When calling this operation all input properties are optional except for the key
that is used to identify the target property. For all other properties you specify the ones that you want to update,
and for those that are to be left unchanged you don’t include them. In case you want to remove a value you would specify the property in question as an empty string.
The following example illustrates an update of an organisation property’s description
, leaving other information unchanged:
{
"key": "vatType",
"description": "The VAT regime to apply."
}
In case you want to altogether remove a value, you specify it as an empty string or array (depending on its type):
{
"key": "vatType",
"allowedValues": [],
"defaultValue": ""
}
The properties that can be updated through this operation are detailed in the payload’s schema.
The operation’s response is empty, signalling through a status 200
(OK) that the update was successfully made.
updateOrganisationProperty - request schema
The payload of the updateOrganisationProperty operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/organisationPropertyInfo_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the createOrganisationProperty and updateOrganisationProperty operation request payloads",
"type": "object",
"properties": {
"key": {
"description": "The unique key used to identify the property.",
"type": "string"
},
"name": {
"description": "The property's name. If not set this will be the same as the property's key.",
"type": "string"
},
"description": {
"description": "The property's description.",
"type": "string"
},
"required": {
"description": "Whether the property must be set before executing test sessions.",
"type": "boolean",
"default": true
},
"editableByUsers": {
"description": "Whether the property can be set by organisation users.",
"type": "boolean",
"default": true
},
"inTests": {
"description": "Whether the property should be exposed to test sessions.",
"type": "boolean",
"default": false
},
"inExports": {
"description": "Whether the property should be included in exports.",
"type": "boolean",
"default": false
},
"inSelfRegistration": {
"description": "Whether the property should be requested during self-registration.",
"type": "boolean",
"default": false
},
"hidden": {
"description": "Whether the property should be hidden from organisation users.",
"type": "boolean",
"default": false
},
"allowedValues": {
"description": "The list of allowed values for the property.",
"type": "array",
"items": {
"$ref": "#/definitions/allowedPropertyValue"
}
},
"displayOrder": {
"description": "The display order of the property when presented to users.",
"type": "number"
},
"dependsOn": {
"description": "The key of another property upon which this property's applicability depends on (see also dependsOnValue).",
"type": "string"
},
"dependsOnValue": {
"description": "If the dependsOn property is set, this is the value that the referred-to property needs to have for this property to be considered applicable.",
"type": "string"
},
"defaultValue": {
"description": "The default value of the property when a new organisation is created.",
"type": "string"
}
},
"required": [
"key"
],
"additionalProperties": false,
"definitions": {
"allowedPropertyValue": {
"description": "An entry for a configuration property representing an allowed value and its display label.",
"type": "object",
"properties": {
"value": {
"description": "The property value.",
"type": "string"
},
"label": {
"description": "The property's display label.",
"type": "string"
}
},
"required": [
"value",
"label"
],
"additionalProperties": false
}
}
}
deleteOrganisationProperty
The deleteOrganisationProperty operation is used to delete the definition of an organisation property. To use it make an HTTP DELETE
to path /api/rest/configure/organisation/{property}
,
setting {property}
to the target property’s key. In addition, you must include in your request an HTTP header named ITB_API_KEY
set to your community API key.
This operation takes no payload when making a request. If the target property has been successfully deleted, it will respond with an
empty body and a status of 200
(OK) to signal that the deletion was successful.
createSystemProperty
The createSystemProperty operation is used to define a new system property for a community. To use it make an HTTP PUT
to path /api/rest/configure/system
and include in your request an HTTP header named ITB_API_KEY
set to your community API key.
In the request’s body you specify the information of the system property, of which the key
is mandatory. The full set of information you can set for the property is defined
in the payload’s schema.
The following example shows how you can create an organisation property with the provided data:
{
"key": "apiEndpoint",
"name": "API endpoint",
"description": "The API endpoint to send messages to.",
"required": true,
"editableByUsers": true,
"inTests": true
}
Other more advanced options include specifying the property’s allowed values, default value, and dependencies. In the latter case, dependsOn
is set with the key of the property
that the current one depends upon:
{
"key": "encrypt",
"name": "Encrypted endpoint",
"description": "Whether the provided endpoint is encrypted.",
"required": true,
"editableByUsers": true,
"inTests": true,
"allowedValues": [
{ "value": "Y", "label": "Yes" },
{ "value": "N", "label": "No" }
],
"defaultValue": "Y",
"dependsOn": "apiEndpoint"
}
Calling this operation, the test bed will create the property and respond with a 200
(OK) response if successful.
createSystemProperty - request schema
The payload of the createSystemProperty operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/systemPropertyInfo_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the createSystemProperty and updateSystemProperty operation request payloads",
"type": "object",
"properties": {
"key": {
"description": "The unique key used to identify the property.",
"type": "string"
},
"name": {
"description": "The property's name. If not set this will be the same as the property's key.",
"type": "string"
},
"description": {
"description": "The property's description.",
"type": "string"
},
"required": {
"description": "Whether the property must be set before executing test sessions.",
"type": "boolean",
"default": true
},
"editableByUsers": {
"description": "Whether the property can be set by organisation users.",
"type": "boolean",
"default": true
},
"inTests": {
"description": "Whether the property should be exposed to test sessions.",
"type": "boolean",
"default": false
},
"inExports": {
"description": "Whether the property should be included in exports.",
"type": "boolean",
"default": false
},
"hidden": {
"description": "Whether the property should be hidden from organisation users.",
"type": "boolean",
"default": false
},
"allowedValues": {
"description": "The list of allowed values for the property.",
"type": "array",
"items": {
"$ref": "#/definitions/allowedPropertyValue"
}
},
"displayOrder": {
"description": "The display order of the property when presented to users.",
"type": "number"
},
"dependsOn": {
"description": "The key of another property upon which this property's applicability depends on (see also dependsOnValue).",
"type": "string"
},
"dependsOnValue": {
"description": "If the dependsOn property is set, this is the value that the referred-to property needs to have for this property to be considered applicable.",
"type": "string"
},
"defaultValue": {
"description": "The default value of the property when a new system is created.",
"type": "string"
}
},
"required": [
"key"
],
"additionalProperties": false,
"definitions": {
"allowedPropertyValue": {
"description": "An entry for a configuration property representing an allowed value and its display label.",
"type": "object",
"properties": {
"value": {
"description": "The property value.",
"type": "string"
},
"label": {
"description": "The property's display label.",
"type": "string"
}
},
"required": [
"value",
"label"
],
"additionalProperties": false
}
}
}
updateSystemProperty
The updateSystemProperty operation is used to update the definition of a system property. To use it make an HTTP POST
to path /api/rest/configure/system
and include in your request an HTTP header named ITB_API_KEY
set to your community API key.
When calling this operation all input properties are optional except for the key
that is used to identify the target property. For all other properties you specify the ones that you want to update,
and for those that are to be left unchanged you don’t include them. In case you want to remove a value you would specify the property in question as an empty string.
The following example illustrates an update of a system property’s description
, leaving other information unchanged:
{
"key": "apiEndpoint",
"description": "The API endpoint root."
}
In case you want to altogether remove a value, you specify it as an empty string or array (depending on its type):
{
"key": "encrypt",
"allowedValues": [],
"defaultValue": ""
}
The properties that can be updated through this operation are detailed in the payload’s schema.
The operation’s response is empty, signalling through a status 200
(OK) that the update was successfully made.
updateSystemProperty - request schema
The payload of the updateSystemProperty operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/systemPropertyInfo_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the createSystemProperty and updateSystemProperty operation request payloads",
"type": "object",
"properties": {
"key": {
"description": "The unique key used to identify the property.",
"type": "string"
},
"name": {
"description": "The property's name. If not set this will be the same as the property's key.",
"type": "string"
},
"description": {
"description": "The property's description.",
"type": "string"
},
"required": {
"description": "Whether the property must be set before executing test sessions.",
"type": "boolean",
"default": true
},
"editableByUsers": {
"description": "Whether the property can be set by organisation users.",
"type": "boolean",
"default": true
},
"inTests": {
"description": "Whether the property should be exposed to test sessions.",
"type": "boolean",
"default": false
},
"inExports": {
"description": "Whether the property should be included in exports.",
"type": "boolean",
"default": false
},
"hidden": {
"description": "Whether the property should be hidden from organisation users.",
"type": "boolean",
"default": false
},
"allowedValues": {
"description": "The list of allowed values for the property.",
"type": "array",
"items": {
"$ref": "#/definitions/allowedPropertyValue"
}
},
"displayOrder": {
"description": "The display order of the property when presented to users.",
"type": "number"
},
"dependsOn": {
"description": "The key of another property upon which this property's applicability depends on (see also dependsOnValue).",
"type": "string"
},
"dependsOnValue": {
"description": "If the dependsOn property is set, this is the value that the referred-to property needs to have for this property to be considered applicable.",
"type": "string"
},
"defaultValue": {
"description": "The default value of the property when a new system is created.",
"type": "string"
}
},
"required": [
"key"
],
"additionalProperties": false,
"definitions": {
"allowedPropertyValue": {
"description": "An entry for a configuration property representing an allowed value and its display label.",
"type": "object",
"properties": {
"value": {
"description": "The property value.",
"type": "string"
},
"label": {
"description": "The property's display label.",
"type": "string"
}
},
"required": [
"value",
"label"
],
"additionalProperties": false
}
}
}
deleteSystemProperty
The deleteSystemProperty operation is used to delete the definition of a system property. To use it make an HTTP DELETE
to path /api/rest/configure/system/{property}
,
setting {property}
to the target property’s key. In addition, you must include in your request an HTTP header named ITB_API_KEY
set to your community API key.
This operation takes no payload when making a request. If the target property has been successfully deleted, it will respond with an
empty body and a status of 200
(OK) to signal that the deletion was successful.
createStatementProperty
The createStatementProperty operation is used to define a new conformance statement (actor) property for a community. To use it make an HTTP PUT
to path /api/rest/configure/actor/{actor}
,
setting {actor}
with the API key of the target specification actor. In addition, you need to include in your request an HTTP header named ITB_API_KEY
set to a community API key.
This API key identifies a community that has access to manage the domain in question, either by being directly linked to it or by being linked to no domain.
In the request’s body you specify the information of the conformance statement property, of which the key
is mandatory. The full set of information you can set for the property is defined
in the payload’s schema.
The following example shows how you can create a conformance statement property with the provided data:
{
"key": "apiEndpoint",
"name": "API endpoint",
"description": "The API endpoint to send messages to.",
"required": true,
"editableByUsers": true,
"inTests": true
}
Other more advanced options include specifying the property’s allowed values, default value, and dependencies. In the latter case, dependsOn
is set with the key of the property
that the current one depends upon:
{
"key": "encrypt",
"name": "Encrypted endpoint",
"description": "Whether the provided endpoint is encrypted.",
"required": true,
"editableByUsers": true,
"inTests": true,
"allowedValues": [
{ "value": "Y", "label": "Yes" },
{ "value": "N", "label": "No" }
],
"defaultValue": "Y",
"dependsOn": "apiEndpoint"
}
Calling this operation, the test bed will create the property and respond with a 200
(OK) response if successful.
createStatementProperty - request schema
The payload of the createStatementProperty operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/statementPropertyInfo_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the createStatementProperty and updateStatementProperty operation request payloads",
"type": "object",
"properties": {
"key": {
"description": "The unique key used to identify the property.",
"type": "string"
},
"name": {
"description": "The property's name. If not set this will be the same as the property's key.",
"type": "string"
},
"description": {
"description": "The property's description.",
"type": "string"
},
"required": {
"description": "Whether the property must be set before executing test sessions.",
"type": "boolean",
"default": true
},
"editableByUsers": {
"description": "Whether the property can be set by organisation users.",
"type": "boolean",
"default": true
},
"inTests": {
"description": "Whether the property should be exposed to test sessions.",
"type": "boolean",
"default": false
},
"inExports": {
"description": "Whether the property should be included in exports.",
"type": "boolean",
"default": false
},
"hidden": {
"description": "Whether the property should be hidden from organisation users.",
"type": "boolean",
"default": false
},
"allowedValues": {
"description": "The list of allowed values for the property.",
"type": "array",
"items": {
"$ref": "#/definitions/allowedPropertyValue"
}
},
"displayOrder": {
"description": "The display order of the property when presented to users.",
"type": "number"
},
"dependsOn": {
"description": "The key of another property upon which this property's applicability depends on (see also dependsOnValue).",
"type": "string"
},
"dependsOnValue": {
"description": "If the dependsOn property is set, this is the value that the referred-to property needs to have for this property to be considered applicable.",
"type": "string"
},
"defaultValue": {
"description": "The default value of the property when a new conformance statement is created.",
"type": "string"
}
},
"required": [
"key"
],
"additionalProperties": false,
"definitions": {
"allowedPropertyValue": {
"description": "An entry for a configuration property representing an allowed value and its display label.",
"type": "object",
"properties": {
"value": {
"description": "The property value.",
"type": "string"
},
"label": {
"description": "The property's display label.",
"type": "string"
}
},
"required": [
"value",
"label"
],
"additionalProperties": false
}
}
}
updateStatementProperty
The updateStatementProperty operation is used to update the definition of a conformance statement (actor) property. To use it make an HTTP POST
to path /api/rest/configure/actor
,
setting {actor}
with the API key of the target specification actor. In addition, you need to include in your request an HTTP header named ITB_API_KEY
set to a community API key.
This API key identifies a community that has access to manage the domain in question, either by being directly linked to it or by being linked to no domain.
When calling this operation all input properties are optional except for the key
that is used to identify the target property. For all other properties you specify the ones that you want to update,
and for those that are to be left unchanged you don’t include them. In case you want to remove a value you would specify the property in question as an empty string.
The following example illustrates an update of a conformance statement property’s description
, leaving other information unchanged:
{
"key": "apiEndpoint",
"description": "The API endpoint root."
}
In case you want to altogether remove a value, you specify it as an empty string or array (depending on its type):
{
"key": "encrypt",
"allowedValues": [],
"defaultValue": ""
}
The properties that can be updated through this operation are detailed in the payload’s schema.
The operation’s response is empty, signalling through a status 200
(OK) that the update was successfully made.
updateStatementProperty - request schema
The payload of the updateStatementProperty operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/statementPropertyInfo_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the createStatementProperty and updateStatementProperty operation request payloads",
"type": "object",
"properties": {
"key": {
"description": "The unique key used to identify the property.",
"type": "string"
},
"name": {
"description": "The property's name. If not set this will be the same as the property's key.",
"type": "string"
},
"description": {
"description": "The property's description.",
"type": "string"
},
"required": {
"description": "Whether the property must be set before executing test sessions.",
"type": "boolean",
"default": true
},
"editableByUsers": {
"description": "Whether the property can be set by organisation users.",
"type": "boolean",
"default": true
},
"inTests": {
"description": "Whether the property should be exposed to test sessions.",
"type": "boolean",
"default": false
},
"inExports": {
"description": "Whether the property should be included in exports.",
"type": "boolean",
"default": false
},
"hidden": {
"description": "Whether the property should be hidden from organisation users.",
"type": "boolean",
"default": false
},
"allowedValues": {
"description": "The list of allowed values for the property.",
"type": "array",
"items": {
"$ref": "#/definitions/allowedPropertyValue"
}
},
"displayOrder": {
"description": "The display order of the property when presented to users.",
"type": "number"
},
"dependsOn": {
"description": "The key of another property upon which this property's applicability depends on (see also dependsOnValue).",
"type": "string"
},
"dependsOnValue": {
"description": "If the dependsOn property is set, this is the value that the referred-to property needs to have for this property to be considered applicable.",
"type": "string"
},
"defaultValue": {
"description": "The default value of the property when a new conformance statement is created.",
"type": "string"
}
},
"required": [
"key"
],
"additionalProperties": false,
"definitions": {
"allowedPropertyValue": {
"description": "An entry for a configuration property representing an allowed value and its display label.",
"type": "object",
"properties": {
"value": {
"description": "The property value.",
"type": "string"
},
"label": {
"description": "The property's display label.",
"type": "string"
}
},
"required": [
"value",
"label"
],
"additionalProperties": false
}
}
}
deleteStatementProperty
The deleteStatementProperty operation is used to delete the definition of a conformance statement (actor) property. To use it make an HTTP DELETE
to path /api/rest/configure/actor/{actor}/{property}
,
setting {actor}
with the API key of the target specification actor, and {property}
with the target property’s key. In addition,
you must include in your request an HTTP header named ITB_API_KEY
set to a community API key.
This API key identifies a community that has access to manage the domain in question, either by being directly linked to it or by being linked to no domain.
This operation takes no payload when making a request. If the target property has been successfully deleted, it will respond with an
empty body and a status of 200
(OK) to signal that the deletion was successful.
Conformance statement management
You can use the test bed’s REST API to manage an organisation’s conformance statements. The following operations are provided:
create: Create a conformance statement linking an organisation’s system with a specification actor.
delete: Delete an organisation’s conformance statement.
report: Produce a conformance statement report for a given conformance statement.
Details on each operation, including sample requests and responses, are provided in the following sections.
create
The create operation is used to create a conformance statement for an organisation, linking one of its systems with a specification
actor. To call the operation make an HTTP PUT
to path /api/rest/conformance/{system}/{actor}
setting the path placeholders as follows:
For
{system}
use the API key of the system you want to create the statement for.For
{actor}
use the API key of the specification actor.
In addition, you must include in your request an HTTP header named ITB_API_KEY
set with the organisation’s API key. Finally, note that
this operation does not take a body.
As an example, if we want to create a conformance statement linking a system and actor with API keys SYSTEM123
and ACTOR456
you
would make a PUT
to /api/rest/conformance/SYSTEM123/ACTOR456
. In terms of response, if the conformance statement was successfully
created you will receive a 200
response with no body.
delete
The delete operation is used to delete an organisation’s conformance statement. To call the operation make an HTTP DELETE
to path /api/rest/conformance/{system}/{actor}
setting the path placeholders as follows:
For
{system}
use the API key of the system that is currently linked to the statement.For
{actor}
use the API key of the relevant specification actor.
In addition, you must include in your request an HTTP header named ITB_API_KEY
set with the organisation’s API key.
As an example, if we want to delete a conformance statement that currently links a system and actor with API keys SYSTEM123
and ACTOR456
you would make a DELETE
to /api/rest/conformance/SYSTEM123/ACTOR456
. In terms of response, if the conformance statement was successfully
removed you will receive a 200
response with no body.
report
The report operation is used to produce a conformance statement report. To use it make an HTTP GET
to path /api/rest/conformance/{system}/{actor}
setting the path placeholders as follows:
For
{system}
use the API key of the system linked to the statement.For
{actor}
use the API key of the relevant specification actor.
By default the format of the returned report will be XML, expressed in the GITB Test Reporting Language (GITB TRL).
You can also request a PDF report by specifying the Accept
HTTP header as application/pdf
. In addition, you must include in your request an additional
HTTP header named ITB_API_KEY
set with the organisation’s API key.
Once this call is made, the test bed will return a response with a 200
status code, whose payload is the report’s content.
The following sample is a complete example of such a report (in the default XML format):
<?xml version="1.0" encoding="UTF-8"?>
<ConformanceStatementReport xmlns="http://www.gitb.com/tr/v1/"
xmlns:ns2="http://www.gitb.com/core/v1/"
xmlns:ns3="http://www.gitb.com/tbs/v1/">
<metadata>
<reportTime>2024-04-02T14:13:18.267+02:00</reportTime>
</metadata>
<statement>
<definition>
<party>
<organisation>
<name>ACME</name>
</organisation>
<system>
<name>Test system</name>
<version>v1.0</version>
<description>A system for test purposes.</description>
</system>
</party>
<domain>
<name>Docs domain</name>
<description>A demo domain for documentation purposes.</description>
</domain>
<specificationGroup>
<name>EU Purchase Order Export Format</name>
<description>Specification to define the data export format for EU Purchase Order Exports.</description>
</specificationGroup>
<specification>
<name>Version 1.02</name>
<description>Version 1.02 of the EU Purchase Order Export Format.</description>
</specification>
<actor>
<name>Actor</name>
<description>Order supplier.</description>
</actor>
</definition>
<summary>
<status>FAILURE</status>
<succeeded>2</succeeded>
<failed>1</failed>
<incomplete>0</incomplete>
</summary>
<lastUpdate>2023-09-29T19:00:01.000+02:00</lastUpdate>
<testOverview>
<testSuite>
<metadata>
<ns2:name>Simple TS</ns2:name>
<ns2:version>0.1</ns2:version>
<ns2:description>Simple test suite.</ns2:description>
</metadata>
<result>FAILURE</result>
<testCases>
<testCase>
<metadata>
<ns2:name>Simple TC</ns2:name>
<ns2:version>1.0</ns2:version>
<ns2:description>A simple test case.</ns2:description>
<ns2:tags>
<ns2:tag name="security" foreground="#ffffff" background="#d20000">Test cases linked to security issues.</ns2:tag>
<ns2:tag name="version 1.0" foreground="#ffffff" background="#000000">Test cases introduced in version 1.02.</ns2:tag>
</ns2:tags>
</metadata>
<result>SUCCESS</result>
<lastUpdate>2023-09-29T19:00:01.000+02:00</lastUpdate>
</testCase>
<testCase>
<metadata>
<ns2:name>Simple TC2</ns2:name>
<ns2:version>1.0</ns2:version>
<ns2:description>A second simple test case.</ns2:description>
</metadata>
<result>FAILURE</result>
<lastUpdate>2023-10-02T17:09:32.000+02:00</lastUpdate>
</testCase>
</testCases>
</testSuite>
<testSuite>
<metadata>
<ns2:name>Simple test suite</ns2:name>
<ns2:version>1.0</ns2:version>
<ns2:description>A simple test suite.</ns2:description>
</metadata>
<result>SUCCESS</result>
<testCases>
<testCase>
<metadata>
<ns2:name>Test case 1</ns2:name>
<ns2:version>1.0</ns2:version>
<ns2:description>A simple test case.</ns2:description>
</metadata>
<result>SUCCESS</result>
<lastUpdate>2023-09-28T15:52:14.000+02:00</lastUpdate>
</testCase>
<testCase optional="true">
<metadata>
<ns2:name>Test case 2</ns2:name>
<ns2:version>1.0</ns2:version>
<ns2:description>Description for the second simple test case.</ns2:description>
</metadata>
<result>SUCCESS</result>
<lastUpdate>2023-09-28T15:52:16.000+02:00</lastUpdate>
</testCase>
</testCases>
</testSuite>
</testOverview>
</statement>
</ConformanceStatementReport>
Domain management
The domain management operations allow you to manage the test bed’s domains, specification groups, specifications and actors. They are useful if you want to have processes external to the test bed manage such information without needing manual actions through the test bed’s user interface. Specifically you can:
All domain management operations use API keys to authorise calls and determine the information to be updated. Two types of API keys are used, depending on the operation:
The key identifying a community, for all operations that a community administrator can do through the user interface.
The master API key, for selected top-level operations that are normally reserved for the overall test bed administrator.
The sections that follow provide instructions and examples for each operation.
createDomain
The createDomain operation is used to create a new domain. To use it make an HTTP PUT
to path /api/rest/domain
and include in your request an HTTP header named ITB_API_KEY
set to your master API key.
In the request’s body you specify the information of the new domain, of which the shortName
and fullName
are mandatory. Other information you
could provide, although not mandatory, would be the domain’s description
and custom reportMetadata
for XML reports.
For the full set of information you can manage check the payload’s schema.
The following example shows how you can create a domain with the provided data:
{
"shortName": "EUPO",
"fullName": "European Purchase Order",
"description": "The European Purchase Order specifications defining the exchange of purchase orders in the EU."
}
Calling this operation, the test bed will create the domain and respond with the domain’s assigned API key:
{
"apiKey": "B277E210X2FB4X4BD7X88B6X951504F45F8F"
}
It is possible to avoid the automatic API key assignment by defining an API key to use as part of the input. This could be useful if you are configuring a test bed instance based on fixed data and you want to ensure assigned API keys have specific, known values.
{
"shortName": "EUPO",
"fullName": "European Purchase Order",
"description": "The European Purchase Order specifications defining the exchange of purchase orders in the EU.",
"apiKey": "A_FIXED_API_KEY_FOR_THE_DOMAIN"
}
In this case the test bed will use the provided API key unless it is found to already exist, in which case a new one will be generated instead. Regardless, the operation will still report the API key that was assigned.
createDomain - request schema
The payload of the createDomain operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/createDomain_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the createDomain operation request payload",
"type": "object",
"properties": {
"shortName": {
"description": "The domain's short name.",
"type": "string"
},
"fullName": {
"description": "The domain's full name.",
"type": "string"
},
"description": {
"description": "The domain's description.",
"type": "string"
},
"reportMetadata": {
"description": "The domain's additional metadata for XML reports.",
"type": "string"
},
"apiKey": {
"description": "The API key to set for the domain. If this key is already assigned, a new one will be generated.",
"type": "string"
}
},
"required": [
"shortName",
"fullName"
],
"additionalProperties": false
}
createDomain - response schema
The payload of the createDomain operation’s response is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/apiKey_response",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema to signal an assigned API key response",
"type": "object",
"properties": {
"apiKey": {
"description": "The API key value.",
"type": "string"
}
},
"required": [
"apiKey"
],
"additionalProperties": false
}
updateDomain
The updateDomain operation is used to update an existing domain. It exists in two variants which differ in how the target domain is identified, the updates that you can do, and the API key you will use for authorisation.
The first variant assumes that you are performing an update as a community administrator would. In this case you can update the domain linked
to the community or any domain, in case the community is not linked to a specific one. The operation is called by making an HTTP POST
to
path /api/rest/domain
and setting an HTTP header named ITB_API_KEY
to your community API key.
The second variant allows you to perform tasks reserved for the test bed administrator. This means that you can manage any domain within the
test bed regardless of the communities linked to it. In this case you call the operation by making an HTTP POST
to path
/api/rest/domain/{domain}
, setting {domain}
to the target domain’s API key. The ITB_API_KEY
HTTP header needs to be set
with the master API key.
When calling this operation, regardless of the specific variant, all input properties are optional. You specify the properties that you want to update, and for the ones that are to be left unchanged you don’t include them. In case you want to remove a value you would specify the property in question as an empty string.
The following example illustrates an update of the domain’s description
, leaving all other information unchanged:
{
"description": "The set of EUPO specifications."
}
In case you want to altogether remove the description, you specify it’s value as an empty string:
{
"description": ""
}
The properties that can be updated through this operation are detailed in the payload’s schema.
The operation’s response is empty, signalling through a status 200
(OK) that the update was successfully made.
updateDomain - request schema
The payload of the updateDomain operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/updateDomain_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the updateDomain operation request payload",
"type": "object",
"properties": {
"shortName": {
"description": "The domain's short name. Skip this if no update should be made.",
"type": "string"
},
"fullName": {
"description": "The domain's full name. Skip this if no update should be made.",
"type": "string"
},
"description": {
"description": "The domain's description. Skip this if no update should be made or provide as an empty string to remove the current value.",
"type": "string"
},
"reportMetadata": {
"description": "The domain's additional metadata for XML reports.",
"type": "string"
}
},
"additionalProperties": false
}
deleteDomain
The deleteDomain operation is used to delete an existing domain. To use it make an HTTP DELETE
to path /api/rest/domain/{domain}
,
setting {domain}
to the target domain’s API key. In addition, you must include in your request an HTTP header named
ITB_API_KEY
set to your master API key.
This operation takes no payload when making a request. If the target domain has been successfully deleted, it will respond with an
empty body and a status of 200
(OK) to signal that the deletion was successful.
createSpecificationGroup
The createSpecificationGroup operation is used to create a new specification group. To use it make an HTTP PUT
to path /api/rest/group
and include in your request an HTTP header named ITB_API_KEY
set to a community API key. This API key identifies the community
that is able to manage the group’s domain, either by being directly linked to it or being linked to no domain.
In the request’s body you specify the information of the new specification group, of which the shortName
and fullName
are mandatory as defined
in the payload’s schema. In case the community identified by the ITB_API_KEY
header
is not linked to a specific domain, you will need to also specify the domain
property with the target domain’s API key.
The following example shows how you can create a specification group with the provided data:
{
"shortName": "Data package",
"fullName": "Data package specification"
}
Calling this operation, the test bed will create the specification group and respond with its assigned API key:
{
"apiKey": "B277E210X2FB4X4BD7X88B6X951504F45F8F"
}
It is possible to avoid the automatic API key assignment by defining an API key to use as part of the input. This could be useful if you are configuring a test bed instance based on fixed data and you want to ensure assigned API keys have specific, known values.
{
"shortName": "Data package",
"fullName": "Data package specification",
"apiKey": "A_FIXED_API_KEY_FOR_THE_GROUP"
}
In this case the test bed will use the provided API key unless it is found to already exist, in which case a new one will be generated instead. Regardless, the operation will still report the API key that was assigned.
createSpecificationGroup - request schema
The payload of the createSpecificationGroup operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/createSpecificationGroup_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the createSpecificationGroup operation request payload",
"type": "object",
"properties": {
"shortName": {
"description": "The group's short name.",
"type": "string"
},
"fullName": {
"description": "The group's full name.",
"type": "string"
},
"description": {
"description": "The group's description.",
"type": "string"
},
"reportMetadata": {
"description": "The group's additional metadata for XML reports.",
"type": "string"
},
"displayOrder": {
"description": "The group's display order relative to other groups and specifications.",
"type": "number"
},
"apiKey": {
"description": "The API key to set for the group. If this key is already assigned, a new one will be generated.",
"type": "string"
},
"domain": {
"description": "The API key of the domain within which to create the group. This can be omitted if the community identified by the community API key used for authorisation is linked to a specific domain.",
"type": "string"
}
},
"required": [
"shortName",
"fullName"
],
"additionalProperties": false
}
createSpecificationGroup - response schema
The payload of the createSpecificationGroup operation’s response is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/apiKey_response",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema to signal an assigned API key response",
"type": "object",
"properties": {
"apiKey": {
"description": "The API key value.",
"type": "string"
}
},
"required": [
"apiKey"
],
"additionalProperties": false
}
updateSpecificationGroup
The updateSpecificationGroup operation is used to update an existing specification group. To use it make an HTTP POST
to path /api/rest/group/{group}
,
setting {group}
to the target specification group’s API key. You also need to include in your request an HTTP header named ITB_API_KEY
set to a community API key. This API key identifies the community that is able to manage the group’s domain, either by being directly linked to it
or being linked to no domain.
When calling this operation all input properties are optional. You specify the properties that you want to update, and for the ones that are to be left unchanged you don’t include them.
The following example illustrates an update of the specification group’s fullName
, leaving other information unchanged:
{
"fullName": "Amazing data package specification"
}
In case you want to altogether remove a property, such as the the group’s description, you specify it’s value as an empty string:
{
"description": ""
}
The properties that can be updated through this operation are detailed in the payload’s schema.
The operation’s response is empty, signalling through a status 200
(OK) that the update was successfully made.
updateSpecificationGroup - request schema
The payload of the updateSpecificationGroup operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/updateSpecificationGroup_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the updateSpecificationGroup operation request payload",
"type": "object",
"properties": {
"shortName": {
"description": "The group's short name. Skip this if no update should be made.",
"type": "string"
},
"fullName": {
"description": "The group's full name. Skip this if no update should be made.",
"type": "string"
},
"description": {
"description": "The group's description. Skip this if no update should be made or provide as an empty string to remove the current value.",
"type": "string"
},
"reportMetadata": {
"description": "The group's additional metadata for XML reports.",
"type": "string"
}
},
"additionalProperties": false
}
deleteSpecificationGroup
The deleteSpecificationGroup operation is used to delete an existing specification group. To use it make an HTTP DELETE
to path /api/rest/group/{group}
,
setting {group}
to the target specification group’s API key. In addition, you must include in your request an HTTP header named
ITB_API_KEY
set to a community API key. This API key identifies the community that is able to manage the group’s domain, either by
being directly linked to it or being linked to no domain.
This operation takes no payload when making a request. If the target specification group has been successfully deleted, it will respond with an
empty body and a status of 200
(OK) to signal that the deletion was successful.
createSpecification
The createSpecification operation is used to create a new specification. To use it make an HTTP PUT
to path /api/rest/specification
and include in your request an HTTP header named ITB_API_KEY
set to a community API key. This API key identifies the community
that is able to manage the specification’s domain, either by being directly linked to it or being linked to no domain.
In the request’s body you specify the information of the new specification, of which the shortName
and fullName
are mandatory as defined
in the payload’s schema. In case the community identified by the ITB_API_KEY
header
is not linked to a specific domain, you will need to also specify the domain
property with the target domain’s API key. Finally, if you
want to place the specification within a specification group, you may also include the group
property set with the group’s API key.
The following example shows how you can create a specification with the provided data:
{
"shortName": "Create order",
"fullName": "Create order specification"
}
In case you use specification groups you could also have referred to the group within which to place this specification:
{
"shortName": "v1.0",
"fullName": "Version 1.0",
"group": "C48CD2F7XB02FX45B5XB483X6DE1294C9F00"
}
Calling this operation, the test bed will create the specification and respond with its assigned API key:
{
"apiKey": "B277E210X2FB4X4BD7X88B6X951504F45F8F"
}
It is possible to avoid the automatic API key assignment by defining an API key to use as part of the input. This could be useful if you are configuring a test bed instance based on fixed data and you want to ensure assigned API keys have specific, known values.
{
"shortName": "Create order",
"fullName": "Create order specification",
"apiKey": "A_FIXED_API_KEY_FOR_THE_SPECIFICATION"
}
In this case the test bed will use the provided API key unless it is found to already exist, in which case a new one will be generated instead. Regardless, the operation will still report the API key that was assigned.
createSpecification - request schema
The payload of the createSpecification operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/createSpecification_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the createSpecification operation request payload",
"type": "object",
"properties": {
"shortName": {
"description": "The specification's short name.",
"type": "string"
},
"fullName": {
"description": "The specification's full name.",
"type": "string"
},
"description": {
"description": "The specification's description.",
"type": "string"
},
"reportMetadata": {
"description": "The specification's additional metadata for XML reports.",
"type": "string"
},
"hidden": {
"description": "Whether the specification should be hidden.",
"type": "boolean",
"default": false
},
"displayOrder": {
"description": "The specification's display order relative to other groups and specifications.",
"type": "number"
},
"apiKey": {
"description": "The API key to set for the specification. If this key is already assigned, a new one will be generated.",
"type": "string"
},
"group": {
"description": "The API key of the specification group within which to create the specification. If not provided the specification will be created directly under the domain.",
"type": "string"
},
"domain": {
"description": "The API key of the domain within which to create the specification. This can be omitted if the community identified by the community API key used for authorisation is linked to a specific domain.",
"type": "string"
}
},
"required": [
"shortName",
"fullName"
],
"additionalProperties": false
}
createSpecification - response schema
The payload of the createSpecification operation’s response is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/apiKey_response",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema to signal an assigned API key response",
"type": "object",
"properties": {
"apiKey": {
"description": "The API key value.",
"type": "string"
}
},
"required": [
"apiKey"
],
"additionalProperties": false
}
updateSpecification
The updateSpecification operation is used to update an existing specification. To use it make an HTTP POST
to path /api/rest/specification/{specification}
,
setting {specification}
to the target specification’s API key. You also need to include in your request an HTTP header named ITB_API_KEY
set to a community API key. This API key identifies the community that is able to manage the specification’s domain, either by being directly linked to it
or being linked to no domain.
When calling this operation all input properties are optional. You specify the properties that you want to update, and for the ones that are to be left unchanged you don’t include them.
The following example illustrates an update of the specification’s fullName
, leaving other information unchanged:
{
"fullName": "Order creation specification"
}
In case you want to altogether remove a property, such as the the specification’s description, you specify it’s value as an empty string:
{
"description": ""
}
You can also use this operation to manage the specification’s grouping. Setting the group
property to a specification group’s API key
will place it within the target group, removing it from its previous group (if defined). If you simply want to remove the specification
from its current group you can specify the group
property as an empty string:
{
"group": ""
}
The properties that can be updated through this operation are detailed in the payload’s schema.
The operation’s response is empty, signalling through a status 200
(OK) that the update was successfully made.
updateSpecification - request schema
The payload of the updateSpecification operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/updateSpecification_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the updateSpecification operation request payload",
"type": "object",
"properties": {
"shortName": {
"description": "The specification's short name. Skip this if no update should be made.",
"type": "string"
},
"fullName": {
"description": "The specification's full name. Skip this if no update should be made.",
"type": "string"
},
"description": {
"description": "The specification's description. Skip this if no update should be made or provide as an empty string to remove the current value.",
"type": "string"
},
"reportMetadata": {
"description": "The specification's additional metadata for XML reports.",
"type": "string"
},
"hidden": {
"description": "Whether the specification should be hidden. Skip this if no update should be made.",
"type": "boolean"
},
"displayOrder": {
"description": "The specification's display order relative to other groups and specifications. Skip this if no update should be made or set to a negative value to remove the ordering altogether.",
"type": "number"
},
"group": {
"description": "The API key of the specification group within which to move the specification. Skip this if no update should be made or provide as an empty string to remove the specification from its current group.",
"type": "string"
}
},
"additionalProperties": false
}
deleteSpecification
The deleteSpecification operation is used to delete an existing specification. To use it make an HTTP DELETE
to path /api/rest/specification/{specification}
,
setting {specification}
to the target specification’s API key. In addition, you must include in your request an HTTP header named
ITB_API_KEY
set to a community API key. This API key identifies the community that is able to manage the specification’s domain, either by
being directly linked to it or being linked to no domain.
This operation takes no payload when making a request. If the target specification has been successfully deleted, it will respond with an
empty body and a status of 200
(OK) to signal that the deletion was successful.
createActor
The createActor operation is used to create a new actor. To use it make an HTTP PUT
to path /api/rest/actor
and include in your request an HTTP header named ITB_API_KEY
set to a community API key. This API key identifies the community
that is able to manage the actor’s domain, either by being directly linked to it or being linked to no domain.
In the request’s body you specify the information of the new actor, of which the identifier
, name
and specification
are mandatory as defined
in the payload’s schema. The specification
property identifies the specification
under which the actor is to be created, and needs to be set with the specification’s API key.
The following example shows how you can create an actor with the provided data:
{
"identifier": "supplier",
"name": "Supplier",
"specification": "1A748C4DXBFD6X4FD3XA196X969D9B695244"
}
Calling this operation, the test bed will create the actor and respond with its assigned API key:
{
"apiKey": "B277E210X2FB4X4BD7X88B6X951504F45F8F"
}
It is possible to avoid the automatic API key assignment by defining an API key to use as part of the input. This could be useful if you are configuring a test bed instance based on fixed data and you want to ensure assigned API keys have specific, known values.
{
"identifier": "supplier",
"name": "Supplier",
"specification": "1A748C4DXBFD6X4FD3XA196X969D9B695244",
"apiKey": "A_FIXED_API_KEY_FOR_THE_ACTOR"
}
In this case the test bed will use the provided API key unless it is found to already exist, in which case a new one will be generated instead. Regardless, the operation will still report the API key that was assigned.
createActor - request schema
The payload of the createActor operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/createActor_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the createActor operation request payload",
"type": "object",
"properties": {
"identifier": {
"description": "The actor's unique identifier in the specification, used to refer to it from test cases.",
"type": "string"
},
"name": {
"description": "The actor's display name.",
"type": "string"
},
"description": {
"description": "The actor's description.",
"type": "string"
},
"reportMetadata": {
"description": "The actor's additional metadata for XML reports.",
"type": "string"
},
"default": {
"description": "Whether the actor should be considered as the default choice for new conformance statements within its specification.",
"type": "boolean",
"default": false
},
"hidden": {
"description": "Whether the actor should be hidden.",
"type": "boolean",
"default": false
},
"displayOrder": {
"description": "The actor's display order relative to other actors when presented in test execution diagrams.",
"type": "number"
},
"apiKey": {
"description": "The API key to set for the actor. If this key is already assigned, a new one will be generated.",
"type": "string"
},
"specification": {
"description": "The API key of the specification within which to create the actor.",
"type": "string"
}
},
"required": [
"identifier",
"name",
"specification"
],
"additionalProperties": false
}
createActor - response schema
The payload of the createActor operation’s response is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/apiKey_response",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema to signal an assigned API key response",
"type": "object",
"properties": {
"apiKey": {
"description": "The API key value.",
"type": "string"
}
},
"required": [
"apiKey"
],
"additionalProperties": false
}
updateActor
The updateActor operation is used to update an existing actor. To use it make an HTTP POST
to path /api/rest/actor/{actor}
,
setting {actor}
to the target actor’s API key. You also need to include in your request an HTTP header named ITB_API_KEY
set to a community API key. This API key identifies the community that is able to manage the actor’s domain, either by being directly linked to it
or being linked to no domain.
When calling this operation all input properties are optional. You specify the properties that you want to update, and for the ones that are to be left unchanged you don’t include them.
The following example illustrates an update of the actor’s name
, leaving other information unchanged:
{
"name": "Order supplier"
}
In case you want to altogether remove a property, such as the the actor’s description, you specify it’s value as an empty string:
{
"description": ""
}
The properties that can be updated through this operation are detailed in the payload’s schema.
The operation’s response is empty, signalling through a status 200
(OK) that the update was successfully made.
updateActor - request schema
The payload of the updateActor operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/updateActor_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the updateActor operation request payload",
"type": "object",
"properties": {
"identifier": {
"description": "The actor's unique identifier in the specification, used to refer to it from test cases. Skip this if no update should be made.",
"type": "string"
},
"name": {
"description": "The actor's display name. Skip this if no update should be made.",
"type": "string"
},
"description": {
"description": "The actor's description. Skip this if no update should be made or provide as an empty string to remove the current value.",
"type": "string"
},
"reportMetadata": {
"description": "The actor's additional metadata for XML reports.",
"type": "string"
},
"default": {
"description": "Whether the actor should be considered as the default choice for new conformance statements within its specification. Skip this if no update should be made.",
"type": "boolean"
},
"hidden": {
"description": "Whether the actor should be hidden. Skip this if no update should be made.",
"type": "boolean"
},
"displayOrder": {
"description": "The actor's display order relative to other actors when presented in test execution diagrams. Skip this if no update should be made or set to a negative value to remove the ordering altogether.",
"type": "number"
}
},
"additionalProperties": false
}
deleteActor
The deleteActor operation is used to delete an existing actor. To use it make an HTTP DELETE
to path /api/rest/actor/{actor}
,
setting {actor}
to the target actor’s API key. In addition, you must include in your request an HTTP header named
ITB_API_KEY
set to a community API key. This API key identifies the community that is able to manage the actor’s domain, either by
being directly linked to it or being linked to no domain.
This operation takes no payload when making a request. If the target actor has been successfully deleted, it will respond with an
empty body and a status of 200
(OK) to signal that the deletion was successful.
Test session management
Apart from launching tests through the user interface you may also launch, manage and report on test sessions using REST API calls. A typical scenario would be to do so as part of a development or quality assurance workflow that would involve the following steps:
Upon changes to your system, or at given intervals, deploy and initialise the latest version of your system.
Once your system is ready, use the test bed’s REST API to launch a series of test sessions for your system.
Have your system proceed, via scripting or responding to test bed requests, to complete the launched test sessions.
Monitor the progress of the launched test sessions by periodically polling the test bed for updates.
Once all test sessions are complete, compile an overview report and shut down your system.
All test session management operations identify relevant data via API keys that are managed as part of the organisation’s details. API keys are defined to cover the following information:
Organisation: The key to identify the specific organisation for which test sessions will be launched or managed.
System: The key to determine the system for which new test sessions are to be launched.
Actor: The key to determine the target specification actor for which to test conformance. Recall that the combination of system and actor essentially define a conformance statement.
Test suite: The key to determine a specific test suite.
Test case: The key to determine a specific test case.
Three operations are made available that allow you to launch, monitor and manage test sessions:
start: Launch one or more test sessions relevant to a given conformance statement.
status: Query the status of one or more test sessions.
stop: Stop one or more test sessions.
All these operations are HTTP calls that include the following:
A HTTP header named
ITB_API_KEY
set with the organisation API key. This header is required to authenticate the request.A JSON payload provided as the body of the request to determine the parameters of the requested action.
Details on each operation, including sample requests and responses, are provided in the following sections.
start
The start operation is used to launch one or more test sessions. You may use the operation’s parameters to specify exactly which test cases to execute, allowing for targetted choices or batch execution of complete sets of tests. You may also define how the selected test cases are launched, by specifying whether they should be parallelised or executed in sequence. In addition, you may provide inputs for the tests to execute that could serve to replace values that would be otherwise provided interactively (e.g. user inputs or uploaded files).
To call the start operation make an HTTP POST
to path /api/rest/tests/start
. As with all test bed REST operations for session
management you must include in your request an HTTP header named ITB_API_KEY
set to your organisation API key.
In the request’s payload you typically define at least the following properties:
The
system
, referring to the API key of the system to be tested. This can be skipped in case the target organisation defines a single system.The
actor
, referring to the API key for the target actor. This can be skipped in case you prefer providing test suite and/or test case identifiers to match the target actor. In any case a single conformance statement must be matched based on the provided inputs.
The operation supports properties testSuite
and testCase
, both provided as arrays including the API keys for specific test suites
and test cases. These properties, in combination with the actor
, define which test cases shall be executed.
For example the following request defines only the actor
, thus launching all test cases defined in the system’s
conformance statement:
{
"system": "B277E210X2FB4X4BD7X88B6X951504F45F8F",
"actor": "28E6E6C9X80BDX40C9XB54DX102800BC32D7"
}
Including in addition the testSuite
property will instruct the test bed to launch the test cases defined in that specific test suite(s):
{
"system": "B277E210X2FB4X4BD7X88B6X951504F45F8F",
"actor": "28E6E6C9X80BDX40C9XB54DX102800BC32D7",
"testSuite": [ "TS1" ]
}
If you want to launch only one or more specific test cases you can use the testCase
property:
{
"system": "B277E210X2FB4X4BD7X88B6X951504F45F8F",
"actor": "28E6E6C9X80BDX40C9XB54DX102800BC32D7",
"testCase": [ "TS1_TC1", "TS1_TC2" ]
}
Apart from selecting the test cases to launch, you may also specify property forceSequentialExecution
to inform the test bed how the
test sessions should be launched. Setting this to true
will force the test bed to launch all tests sequentially. By default, this is
considered as false
, meaning that the test bed will launch all test sessions in parallel, unless certain of the selected test cases
require sequential execution.
{
"system": "B277E210X2FB4X4BD7X88B6X951504F45F8F",
"actor": "28E6E6C9X80BDX40C9XB54DX102800BC32D7",
"forceSequentialExecution": true
}
As a complement to the information on which tests are to be launched, you may also provide one or more inputs for the selected test cases. These inputs will be added to the test session context before executing each test, overriding any variables defined with default values. Providing inputs can be very useful allowing you to execute tests that would otherwise require user interactions (which in this case will be skipped).
Note
Declaring input variables in test cases: If you design your test cases based on REST API inputs you will also need to declare the inputs as test case variables.
The inputs provided can be done so in a flexible manner, defining each input (e.g. a text or even a file) once and mapping it to the test cases
for which it should be considered. To do this you use the inputMapping
property, an array with one item per input, complemented by the
information on the test cases to apply it to. Regarding this test case mapping, you may specify property testSuite
to map it to the tests
of certain test suites, property testCase
to map it to certain test cases, or skip these altogether to apply it to all tests.
For example, the following request defines an input named “countryCode” that applies to all test cases, and a second input named “partyId” that only applies to two specific ones:
{
"system": "B277E210X2FB4X4BD7X88B6X951504F45F8F",
"actor": "28E6E6C9X80BDX40C9XB54DX102800BC32D7",
"inputMapping": [
{
"input": {
"name": "countryCode",
"value": "BE"
}
},
{
"testCase": ["TS1_TC1", "TS1_TC2"],
"input": {
"name": "partyId",
"value": "ID12345"
}
}
]
}
The definition of each input
property is quite flexible, allowing you to define complete files as well as complex structures such as maps.
To define a file you would including its content as a Base64-encoded string, setting appropriately the embeddingMethod
and type
properties
on its relevant input:
{
"system": "B277E210X2FB4X4BD7X88B6X951504F45F8F",
"actor": "28E6E6C9X80BDX40C9XB54DX102800BC32D7",
"inputMapping": [
{
"testCase": ["TS1_TC1"],
"input": {
"name": "aFile",
"embeddingMethod": "BASE64",
"type": "binary",
"value": "ZGY6TEtNZmRzYSdrZ2ptZmdobDthZyBcb2VrZ2hhc......"
}
}
]
}
When providing a map as an input you do so by including its entries within the top-level map input, in its item
property:
{
"system": "B277E210X2FB4X4BD7X88B6X951504F45F8F",
"actor": "28E6E6C9X80BDX40C9XB54DX102800BC32D7",
"inputMapping": [
{
"testCase": ["TS1_TC1"],
"input": {
"name": "countryInfo",
"type": "map",
"item": [
{ "name": "countryCode", "value": "BE" },
{ "name": "countryName", "value": "Belgium" }
]
}
}
]
}
For the full specification of the start operation’s request payload you may check its JSON schema definition.
The response you receive from the start operation, includes a confirmation of the test sessions that have been started or planned for execution
(if execution was requested to be sequential). The information for each scheduled session is returned in the createdSessions
array, of which
each item corresponds to one session. For each session you are informed of its relevant testSuite
and testCase
, as well as its assigned
session
identifier with which you can follow its progress.
{
"createdSessions": [
{
"testSuite": "TS1",
"testCase": "TS1_TC1",
"session": "63b76ce6-5ade-431f-8620-8dadb13d2f42"
},
{
"testSuite": "TS1",
"testCase": "TS1_TC2",
"session": "a866297c-ccb0-4133-9ae9-2c3af7aba0bd"
}
]
}
You may use the reported session identifiers to check the sessions’ status and, if needed, forcibly stop them.
start - request schema
The payload of the start operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/start_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the tests' start operation request payload",
"type": "object",
"properties": {
"system": {
"description": "The API KEY identifying the target system. This can be skipped in case the target organisation defines a single system.",
"type": "string"
},
"actor": {
"description": "The API KEY identifying the target actor. This can be skipped in case you prefer providing test suite and/or test case identifiers to match the target actor. In any case a single conformance statement must be matched based on the provided inputs.",
"type": "string"
},
"forceSequentialExecution": {
"description": "Whether the test sessions should be forced to execute sequentially.",
"type": "boolean",
"default": false
},
"testSuite": {
"description": "The identifier(s) of the test suites to execute.",
"type": "array",
"items": {
"type": "string"
}
},
"testCase": {
"description": "The identifier(s) of the test cases to execute.",
"type": "array",
"items": {
"type": "string"
}
},
"inputMapping": {
"description": "Inputs to provide to test sessions. These can be applied to all test sessions, or certain test sessions identified by their relevant test case or test suite identifiers.",
"type": "array",
"items": {
"$ref": "#/definitions/inputMapping"
}
}
},
"additionalProperties": false,
"definitions": {
"inputMapping": {
"description": "Data relevant to an input provided to create one or more test sessions.",
"type": "object",
"properties": {
"testSuite": {
"description": "The identifiers of the test suite for which this input is to be provided.",
"type": "array",
"items": {
"type": "string"
}
},
"testCase": {
"description": "The identifiers of the test case for which this input is to be provided.",
"type": "array",
"items": {
"type": "string"
}
},
"input": {
"$ref": "#/definitions/anyContent"
}
},
"required": [
"input"
],
"additionalProperties": false
},
"anyContent": {
"description": "The data and metadata relevant to a given test session input.",
"type": "object",
"properties": {
"name": {
"description": "The name of the input through which it is referenced in test cases.",
"type": "string"
},
"embeddingMethod": {
"description": "The way in which the value of the input is to be interpreted (as the actual string value, as a Base64-encoded string or as a remote URI location).",
"type": "string",
"enum": [
"STRING",
"BASE64",
"URI"
],
"default": "STRING"
},
"value": {
"description": "The value of the input provided as a string, to be interpreted based on the embeddingMethod input. This may be missing in case of a complex type (list or map).",
"type": "string"
},
"type": {
"description": "The data type of this input.",
"type": "string",
"enum": [
"string",
"number",
"boolean",
"binary",
"object",
"schema",
"map",
"list"
],
"default": "string"
},
"encoding": {
"type": "string"
},
"item": {
"description": "In case this is a complex data type (list or map) this is the array of child (contained) types.",
"type": "array",
"items": {
"$ref": "#/definitions/anyContent"
}
}
},
"additionalProperties": false
}
}
}
start - response schema
The payload of the start operation’s response is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/start_response",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the tests' start operation response payload",
"type": "object",
"properties": {
"createdSessions": {
"description": "Information on the created test session(s).",
"type": "array",
"items": {
"$ref": "#/definitions/sessionCreationInformation"
}
}
},
"required": [
"createdSessions"
],
"additionalProperties": false,
"definitions": {
"sessionCreationInformation": {
"description": "The metadata for a created test session.",
"type": "object",
"properties": {
"testSuite": {
"description": "The identifier of the test session's relevant test suite.",
"type": "string"
},
"testCase": {
"description": "The identifier of the test session's relevant test case.",
"type": "string"
},
"session": {
"description": "The identifier assigned to the test session.",
"type": "string"
}
},
"required": [
"testSuite",
"testCase",
"session"
],
"additionalProperties": false
}
}
}
status
The status operation is used to check the progress of one or more specific test sessions. It can be used with any test session, not only sessions launched via the test bed’s REST API, as long as you are authorised to view them.
To call the status operation make an HTTP POST
to path /api/rest/tests/status
. As with all test bed REST operations for session
management you must include in your request an HTTP header named ITB_API_KEY
set to your organisation API key.
Note
Using GET: Prior to release 1.17.0 the status operation was available through HTTP GET
. This remains possible as an alternative
to POST
but is not part of the API’s OpenAPI documentation as GET
requests are not supposed to
have body content.
In the request’s payload you may provide two properties to define your query:
The
session
array, including one or more session identifiers to look up.The
withLogs
boolean flag to specify whether you want to view the detailed log trace for each returned session. By default log traces are not returned, but you can set this totrue
to include them.The
withReports
boolean flag to specify whether you want to also include the sessions’ XML report expressed in the GITB Test Reporting Language (GITB TRL). By default reports are not included.
The following example call makes a query for one test session, choosing to also return its detailed log:
{
"session": ["08e49917-d560-4ffb-bbf5-280bf1084148"],
"withLogs": true
}
As a response for the status operation, the test bed returns the latest information for the requested sessions in an array named sessions
.
This includes one item per reported session which includes in turn the following properties:
session
, for the session’s identifier.result
, one of “SUCCESS”, “FAILURE” or “UNDEFINED” for the overall test status.startTime
, containing a timestamp for the session’s launch time.
The above properties are included for all test sessions, active or completed. If a session is completed this information additionally includes the following properties:
endTime
, containing a timestamp of the session’s completion time.message
, optionally included if an overall output message was produced by the test session.
In case detailed log traces were requested (i.e. property withLogs
was included and set to true
), each test session will
also include a property named logs
. This is a string array containing one item per reported log message. Similarly, if
test session reports were requested (i.e. property withReports
was included and set to true
), a further property named
report
will be included. This is a string value that includes the complete XML content of the report as a JSON-escaped string
(click here
for a complete XML report sample).
The following example illustrates the status information returned for a single completed test session with logs and reports included:
{
"sessions": [
{
"session": "08e49917-d560-4ffb-bbf5-280bf1084148",
"result": "FAILURE",
"startTime": "2022-03-17T13:28:16Z",
"endTime": "2022-03-17T13:28:38Z",
"message": "Your query did not have the expected type.",
"logs": [
"[2022-03-17 14:28:15] DEBUG - Configuring session [08e49917-d560-4ffb-bbf5-280bf1084148]",
"[2022-03-17 14:28:15] INFO - Starting session",
"[2022-03-17 14:28:15] DEBUG - Status update - step [Query system] - ID [1]: PROCESSING",
"[2022-03-17 14:28:15] DEBUG - Status update - step [Query system] - ID [1]: WAITING",
"[2022-03-17 14:28:15] WARN - Received 'receive' call from Test Bed",
"[2022-03-17 14:28:37] DEBUG - Received notification",
"[2022-03-17 14:28:37] DEBUG - Status update - step [Query system] - ID [1]: COMPLETED",
"[2022-03-17 14:28:37] DEBUG - Status update - step [Response] - ID [2]: PROCESSING",
"[2022-03-17 14:28:37] DEBUG - Status update - step [Response] - ID [2]: COMPLETED",
"[2022-03-17 14:28:37] DEBUG - Status update - step [Sequence] - ID [3]: PROCESSING",
"[2022-03-17 14:28:37] DEBUG - Status update - step [Verify the query type] - ID [3.1]: PROCESSING",
"[2022-03-17 14:28:37] DEBUG - Status update - step [Verify the query type] - ID [3.1]: ERROR",
"[2022-03-17 14:28:37] DEBUG - Status update - step [Sequence] - ID [3]: ERROR",
"[2022-03-17 14:28:37] DEBUG - Status update - step [Call] - ID [3]: ERROR",
"[2022-03-17 14:28:37] DEBUG - Preparing to stop",
"[2022-03-17 14:28:38] INFO - Session finished with result [ERROR]"
],
"report": "<?xml version=\"1.0\"><TestCaseOverviewReport>...</TestCaseOverviewReport>"
}
]
}
status - request schema
The payload of the status operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/status_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the tests' status operation request payload",
"type": "object",
"properties": {
"session": {
"description": "The session identifier(s) to look up.",
"type": "array",
"items": {
"type": "string"
}
},
"withLogs": {
"description": "Whether or not the log output for each session should be returned.",
"type": "boolean",
"default": false
},
"withReports": {
"description": "Whether or not the XML test case report should be returned.",
"type": "boolean",
"default": false
}
},
"additionalProperties": false,
"required": [
"session"
]
}
status - response schema
The payload of the status operation’s response is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/status_response",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the tests' status operation response payload",
"type": "object",
"properties": {
"sessions": {
"description": "The list of status information for retrieved test sessions.",
"type": "array",
"items": {
"$ref": "#/definitions/sessionStatus"
}
}
},
"required": [
"sessions"
],
"additionalProperties": false,
"definitions": {
"sessionStatus": {
"description": "Status information for a test session.",
"type": "object",
"properties": {
"session": {
"description": "The session's unique identifier.",
"type": "string"
},
"result": {
"description": "The session's result.",
"type": "string",
"enum": [
"SUCCESS",
"FAILURE",
"UNDEFINED"
]
},
"startTime": {
"description": "The session start time.",
"type": "string",
"format": "yyyy-MM-ddTHH:mm:ssZ"
},
"endTime": {
"description": "The session end time (if the session is completed).",
"type": "string",
"format": "yyyy-MM-ddTHH:mm:ssZ"
},
"message": {
"description": "The resulting output message of the session.",
"type": "string"
},
"logs": {
"description": "The list of log entries produced by the session.",
"type": "array",
"items": {
"type": "string"
}
},
"report": {
"description": "The test session's XML report.",
"type": "string"
}
},
"required": [
"session",
"result",
"startTime"
],
"additionalProperties": false
}
}
}
stop
The stop operation is used to forcibly terminate one or more specific test sessions. It can be used with any test session, not only sessions launched via the test bed’s REST API, as long as you are authorised to view them.
To call the stop operation make an HTTP POST
to path /api/rest/tests/stop
. As with all test bed REST operations for session
management you must include in your request an HTTP header named ITB_API_KEY
set to your organisation API key.
In the request’s payload you are expected to provide an array named session
, including the session identifiers for one or more test sessions
you want to stop. In the following example, a request is being made to terminate two test sessions:
{
"session": ["08e49917-d560-4ffb-bbf5-280bf1084148", "a866297c-ccb0-4133-9ae9-2c3af7aba0bd"]
}
Once this call is made, the test bed will immediately terminate the requested test sessions. The response to the stop operation has an
empty body and is returned with a 200
(OK) status code.
stop - request schema
The payload of the stop operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/stop_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the tests' stop operation request payload",
"type": "object",
"properties": {
"session": {
"description": "The identifier(s) of the test session(s) to stop.",
"type": "array",
"items": {
"description": "Test session identifier.",
"type": "string"
}
}
},
"required": [
"session"
],
"additionalProperties": false
}
report
The report operation is used to retrieve a test session’s report. It can be used with any test session, not only sessions launched via the test bed’s REST API, as long as you are authorised to view them.
To call the report operation make an HTTP GET
to path /api/rest/tests/report/{sessionId}
, where sessionId
is replaced by the session’s identifier.
As with all test bed REST operations for session management you must include in your request an HTTP header named ITB_API_KEY
set to your organisation API key.
The format of the report is by default XML, using in particular the GITB Test Reporting Language (GITB TRL) syntax.
You may also request the report in PDF, by setting the Accept
HTTP header to application/pdf
.
Once this call is made, the test bed will return a response with a 200
(OK) status code, whose payload is the report’s content. The following sample is a complete
example of such a report (in the default XML format):
<?xml version="1.0" encoding="UTF-8" standalone="yes"?>
<TestCaseOverviewReport xmlns="http://www.gitb.com/tr/v1/" xmlns:ns2="http://www.gitb.com/core/v1/" xmlns:ns3="http://www.gitb.com/tbs/v1/" id="UBL_invoice_validation_test_3">
<metadata>
<ns2:name>TC3: Upload minimal invoice</ns2:name>
<ns2:description>Test case to verify the correctness of a minimal UBL invoice. The invoice is provided manually through user upload.</ns2:description>
</metadata>
<startTime>2022-10-14T15:21:14.000+02:00</startTime>
<endTime>2022-10-14T15:21:31.000+02:00</endTime>
<result>FAILURE</result>
<message>The provided invoice failed validation. Check the failed validation step(s) for further details.</message>
<steps>
<step id="1">
<description>Step 1: UBL invoice upload</description>
<report xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:type="TAR" id="1">
<date>2022-10-14T15:21:25.767+02:00</date>
<result>SUCCESS</result>
</report>
</step>
<step id="2">
<description>Step 2: Validate invoice against UBL 2.1 Invoice Schema</description>
<report xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:type="TAR" name="XML Schema Validation" id="2">
<date>2022-10-14T15:21:25.853+02:00</date>
<result>SUCCESS</result>
<counters>
<nrOfAssertions>0</nrOfAssertions>
<nrOfErrors>0</nrOfErrors>
<nrOfWarnings>0</nrOfWarnings>
</counters>
<reports/>
</report>
</step>
<step id="3">
<description>Step 3: Validate invoice against BII2 CORE restrictions for Invoice Transaction</description>
<report xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:type="TAR" name="Schematron Validation" id="3">
<date>2022-10-14T15:21:29.756+02:00</date>
<result>SUCCESS</result>
<counters>
<nrOfAssertions>0</nrOfAssertions>
<nrOfErrors>0</nrOfErrors>
<nrOfWarnings>1</nrOfWarnings>
</counters>
<reports>
<warning xsi:type="BAR">
<description>Attribute '@listID' marked as not used in the given context.</description>
<location>xml:12:0</location>
</warning>
</reports>
</report>
</step>
<step id="4">
<description>Step 4: Validate invoice against BII RULES</description>
<report xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:type="TAR" name="Schematron Validation" id="4">
<date>2022-10-14T15:21:30.250+02:00</date>
<result>FAILURE</result>
<counters>
<nrOfAssertions>0</nrOfAssertions>
<nrOfErrors>2</nrOfErrors>
<nrOfWarnings>0</nrOfWarnings>
</counters>
<reports>
<error xsi:type="BAR">
<description>[BII2-T10-R051]-Sum of line amounts MUST equal the invoice line net amounts</description>
<location>xml:172:0</location>
</error>
<error xsi:type="BAR">
<description>[BII2-T10-R052]-An invoice total without VAT MUST equal the sum of line amounts plus the sum of charges on document level minus the sum of allowances on document level</description>
<location>xml:172:0</location>
</error>
</reports>
</report>
</step>
</steps>
</TestCaseOverviewReport>
Test suite management
The test bed foresees API operations to deploy and undeploy test suites. Managing test suites in this way is primarily used during test suite development, to allow the deployment of test suites via automation processes.
Test suite management operations make use of API keys to determine the information relevant to a specific call. These keys are:
The key to identify a specification, displayed when viewing a specification’s details.
The identifier of a test suite, displayed when viewing a test suite’s details.
The API includes the following operations to manage test suites:
deploy: Deploy a (non-shared) test suite to a specification.
undeploy: Remove a (non-shared) test suite from a specification.
deployShared: Deploy a shared test suite to a community’s domain.
undeployShared: Remove a shared test suite from a community’s domain.
linkShared: Link a shared test suite to one or more specifications.
unlinkShared: Unlink a shared test suite from one or more specifications.
Details on each operation, including sample requests and responses, are provided in the following sections.
deploy
The deploy operation is used to add a new or updated test suite to a specification. Apart from providing the test suite itself, the operation’s parameters allow you to specify how to handle validation issues and existing conformance tests.
To call the deploy operation make an HTTP POST
to path /api/rest/testsuite/deploy
. To authorise the operation and identify the specification domain
to be updated, you must include in your request an HTTP header named ITB_API_KEY
set to your community API key.
In the request’s payload you will need to define at least the following information:
The
specification
, referring to the API key of the specification to be updated.The
testSuite
, including the data of the test suite archive being deployed.
Apart from these properties, you may optionally specify additional properties as boolean flags to determine archive handling options. Specifically:
Set
ignoreWarnings
totrue
to allow the test suite’s deployment even in case of validation warnings. By default validation warnings will prevent the deployment from completing.Set
replaceTestHistory
totrue
to clear any existing conformance testing history relevant to the test suite. By default existing tests are maintained.Set
updateSpecification
totrue
to update existing specification metadata to values provided in the test suite archive. By default existing information will not be updated.
In addition to the above properties, you may also specify an array named testCases
that provides fine-grained instructions on how to handle the test suite’s
test cases if these are found to already exist. For test cases not specified in this way, or if the testCases
array is altogether missing, the values provided
in replaceTestHistory
and updateSpecification
are treated as the defaults. The items of the testCases
array define the following properties:
identifier
, referring to the identifier of the test case.updateSpecification
, which can be set totrue
to update the test case’s metadata (name and description).replaceTestHistory
, which can be set totrue
to reset the testing history for the test case.
The deploy operation actually comes with two variants to allow you to provide the test suite archive in the way that best fits your needs. The selected
approach is determined by you by setting the request’s Content-Type
header to match the type of submission you are making. Specifically:
Setting
Content-Type
toapplication/json
will consider that the request’s body is JSON that includes the test suite archive as a BASE64 encoded string. The request’s inputs in this case are added as JSON properties with the BASE64 encoded string added as propertytestSuite
.Setting
Content-Type
tomultipart/form-data
will consider the request as a multipart form submission. The request’s inputs in this case will be request parameters with the test suite archive namedtestSuite
(the request’s file part). In addition, thetestCases
array is replaced in this case by four repeatable parameters namedtestCaseWithSpecificationUpdate
,testCaseWithoutSpecificationUpdate
,testCaseWithTestHistoryReplacement
andtestCaseWithoutTestHistoryReplacement
, each set with the relevant test case identifier.
The approach to follow depends on the client tool you want to use to make the calls. When submitting as JSON you will need to always calculate the BASE64 string of the test suite archive before
including it in the payload. In contrast, if you make a multipart form submission, your tool should be able to correctly construct a multipart request with the relevant part
boundaries. If you use a tool such as curl
that handles this, the multipart form approach is likely simpler as you can simply point to the archive’s file to submit without
using additional tools to generate BASE64 strings.
The following sample is a JSON request to deploy a test suite to a specification (the test suite’s BASE64 encoded string is truncated for brevity).
{
"specification": "B277E210X2FB4X4BD7X88B6X951504F45F8F",
"testSuite": "UEsDBBQAAAAIAIWIr...wNAAAAAA=="
}
As discussed earlier you may also include additional flags to determine how the upload should be handled. The following example includes the
ignoreWarnings
, replaceTestHistory
and updateSpecification
flags to override the default behaviours.
{
"specification": "B277E210X2FB4X4BD7X88B6X951504F45F8F",
"ignoreWarnings": true,
"replaceTestHistory": true,
"updateSpecification": true,
"testSuite": "UEsDBBQAAAAIAIWIr...wNAAAAAA=="
}
For the full specification of the deploy operation’s request payload, when this is provided as JSON, you may check its JSON schema definition.
Once the deploy operation has completed you receive a JSON response to notify you of the deployment’s result. This response will always include
a boolean completed
flag to inform you whether the deployment was actually carried out. Alongside this you may optionally receive report items
produced by the test suite’s validation in three arrays named errors
, warnings
and messages
. Each item of these arrays includes the finding’s description
and location
, the latter being the path of the test suite’s resource (e.g. a test case file) that resulted in it being reported. A test suite’s
deployment may not be completed in case it’s validation produced errors or warnings (that were not set to be ignored via the request’s ignoreWarnings
flag).
Besides the overall status and validation summary, the response will also include the API keys of all data created, or affects by the test suite. These keys allow you to automate other operations related to this test suite through the REST API, such as running test sessions or managing conformance statements. The returned API keys include:
The identifier of the test suite that was created or updated as a result of the operation.
The identifiers of test cases that form the latest version of the test suite.
The names and identifiers of the target specifications.
The names and identifiers of the test suite actors.
The following example presents a response that produced a validation warning but was nonetheless completed:
{
"completed": true,
"warnings": [
{
"description": "[TDL-015] Actor [Actor2] is not referenced in any test cases."
}
],
"identifiers": {
"testSuite": "testSuite1",
"testCases": [ "testCase1", "testCase2", "testCase3" ],
"specifications": [
{
"name": "Specification 1",
"identifier": "77040396X168EX40CDXBD3FX62347E1A09E6",
"actors": [
{
"name": "Actor 1",
"identifier": "28E6E6C9X80BDX40C9XB54DX102800BC32D7"
},
{
"name": "Actor 2",
"identifier": "4F5E9DEBX1F5DX4ECBX92DBXC5DEF1035643"
}
]
}
]
}
}
For the full specification of the deploy operation’s response payload you may check its JSON schema definition.
deploy - request schema (JSON case)
The payload of the deploy operation’s request (in the case it submitted as JSON content) is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/deploy_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the test suites' deploy operation request payload",
"type": "object",
"properties": {
"testSuite": {
"description": "The shared test suite archive to deploy provided as a Base64-encoded string.",
"type": "string",
"format": "base64"
},
"testCases": {
"description": "Update choices for specific test cases.",
"type": "array",
"items": {
"$ref": "#/definitions/testCaseDeploymentAction"
}
},
"specification": {
"description": "The API KEY identifying the target specification.",
"type": "string"
},
"ignoreWarnings": {
"description": "Whether test suite validation warnings should be ignored.",
"type": "boolean",
"default": false
},
"replaceTestHistory": {
"description": "Whether the test history linked to the test suite's test cases should be reset. This is the default setting to consider if no test case specific options are defined.",
"type": "boolean",
"default": false
},
"updateSpecification": {
"description": "Whether the specification's metadata should be updated using the provided test suite's content. For test cases' metadata this setting will be considered as the default, if no test case specific options are defined.",
"type": "boolean",
"default": false
}
},
"required": [ "specification", "testSuite" ],
"additionalProperties": false,
"definitions": {
"testCaseDeploymentAction": {
"description": "Deployment choices for a given test case.",
"type": "object",
"properties": {
"identifier": {
"description": "The test case identifier.",
"type": "string"
},
"updateSpecification": {
"description": "Whether the metadata for this test case should be updated.",
"type": "boolean",
"default": false
},
"replaceTestHistory": {
"description": "Whether the testing history for this test case should be reset.",
"type": "boolean",
"default": false
}
}
}
}
}
deploy - response schema
The payload of the deploy operation’s response is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/deploy_response",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the test suites' deploy operation response payload",
"type": "object",
"properties": {
"completed": {
"description": "Whether or not the deployment was completed.",
"type": "boolean"
},
"errors": {
"description": "The list of errors resulting from the test suite's validation.",
"type": "array",
"items": {
"$ref": "#/definitions/testSuiteValidationReportItem"
}
},
"warnings": {
"description": "The list of warnings resulting from the test suite's validation.",
"type": "array",
"items": {
"$ref": "#/definitions/testSuiteValidationReportItem"
}
},
"messages": {
"description": "The list of information messages resulting from the test suite's validation.",
"type": "array",
"items": {
"$ref": "#/definitions/testSuiteValidationReportItem"
}
},
"identifiers": {
"description": "The API key identifiers linked to the test suite.",
"type": "object",
"required": [
"testSuite"
],
"properties": {
"testSuite": {
"description": "The test suite identifier.",
"type": "string"
},
"testCases": {
"description": "The identifiers of the test suite's test cases.",
"type": "array",
"items": {
"type": "string"
}
},
"specifications": {
"description": "The identifiers linked to the test suite's linked specification(s).",
"type": "array",
"items": {
"type": "object",
"required": [
"name",
"identifier"
],
"properties": {
"name": {
"description": "The specification's name.",
"type": "string"
},
"identifier": {
"description": "The specification's API key.",
"type": "string"
},
"actors": {
"description": "The information for the actors linked to the specification.",
"type": "array",
"items": {
"$ref": "#/definitions/testSuiteUploadActorKeys"
}
}
}
}
}
}
}
},
"required": [
"completed"
],
"additionalProperties": false,
"definitions": {
"testSuiteValidationReportItem": {
"description": "A test suite validation report finding.",
"type": "object",
"properties": {
"description": {
"description": "The finding's description.",
"type": "string"
},
"location": {
"description": "The path of the resource within the test suite that resulted in this finding.",
"type": "string"
}
},
"required": [
"description"
],
"additionalProperties": false
},
"testSuiteUploadActorKeys": {
"description": "The API keys for the actors related to the test suite.",
"type": "object",
"required": [
"name",
"identifier"
],
"properties": {
"name": {
"description": "The name of the actor.",
"type": "string"
},
"identifier": {
"description": "The API key of the actor.",
"type": "string"
}
}
}
}
}
undeploy
The undeploy operation is used to remove a test suite from a specification. Removing a test suite will result in the relevant conformance testing history being cleared.
To call the undeploy operation make an HTTP POST
to path /api/rest/testsuite/undeploy
. To authorise the operation and identify the specification domain
from which the test suite will be removed, you must include in your request an HTTP header named ITB_API_KEY
set to your community API key.
In the request’s payload you will need to define the following two properties:
The
specification
, referring to the API key of the specification to be updated.The
testSuite
, referring to the identifier of the test suite to be removed.
The following sample is a request to remove a test suite from a specification.
{
"specification": "B277E210X2FB4X4BD7X88B6X951504F45F8F",
"testSuite": "test_suite_1"
}
Once this call is made, the test bed will remove the specified test suite and clear any related conformance tests. The response to the undeploy operation has an
empty body and is returned with a 200
(OK) status code.
undeploy - request schema
The payload of the undeploy operation’s request is defined by the following JSON Schema
:
{
"$id": "https://www.itb.ec.europa.eu/api/undeploy_request",
"$schema": "http://json-schema.org/draft-07/schema#",
"description": "JSON schema for the test suites' undeploy operation request payload",
"type": "object",
"properties": {
"specification": {
"description": "The API key value to uniquely identify the specification from which the test suite will be undeployed from.",
"type": "string"
},
"testSuite": {
"description": "The identifier of the test suite that will be undeployed.",
"type": "string"
}
},
"required": [
"specification",
"testSuite"
],
"additionalProperties": false
}
OpenAPI documentation
The test bed’s REST API is also documented using the standard OpenAPI specification. You may
download this from here
, or access it live from the test bed from path /api/rest
. On a typical
developer instance this would be available at http://localhost:9000/api/rest
.
The API’s documentation does not only provide a standardised representation of its operations. It also allows it to be imported into tools that can process it to generate code, documentation and even call the live services.
An example of a popular, free and open-source tool for this purpose is the Swagger UI which provides a full user interface to explore and use an API. This can either be downloaded or used directly from the cloud. If you use Docker and have it installed on your workstation you can get it up and running by issuing:
docker pull swaggerapi/swagger-ui
docker run -p 80:8080 swaggerapi/swagger-ui
Executing the above two commands will download and launch Swagger UI, making it available at http://localhost
. Accessing this
you may now view and use the test bed’s REST API:
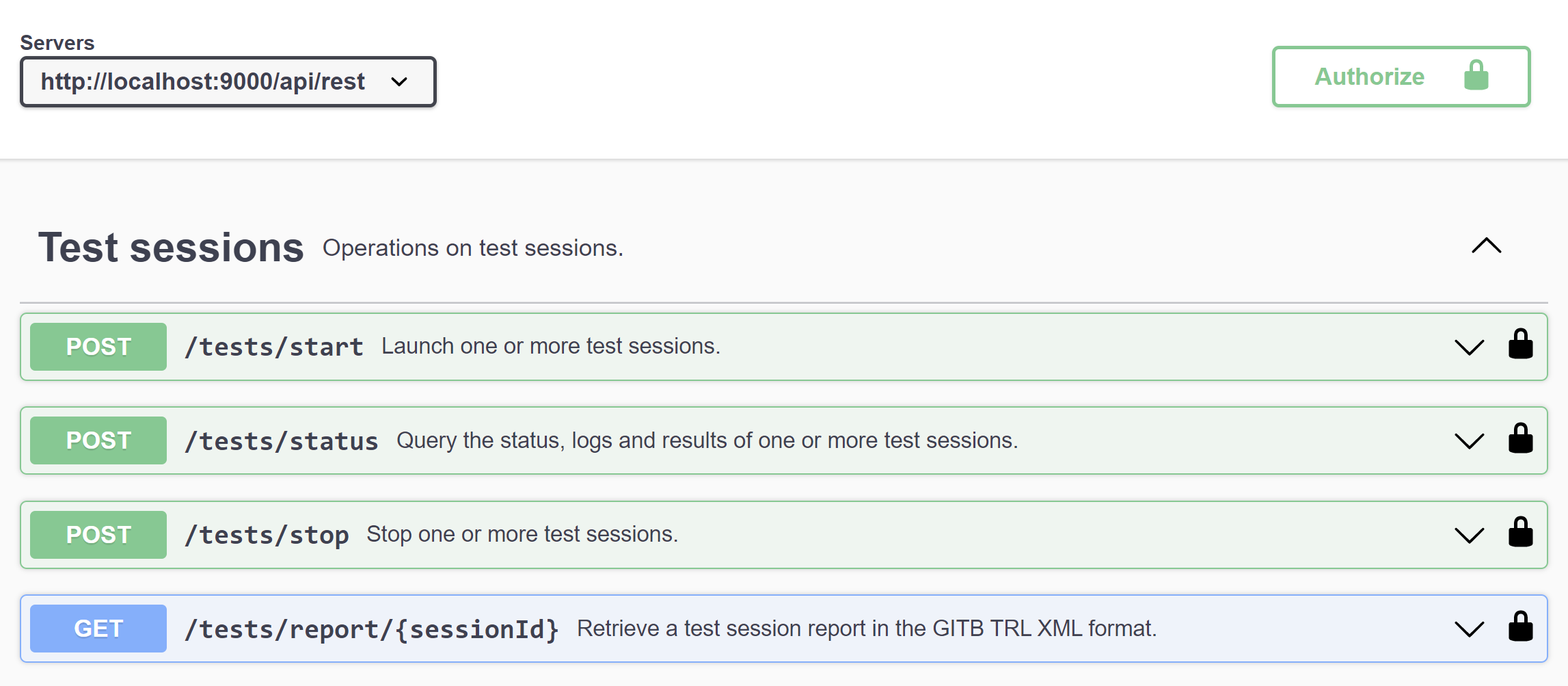
Health monitoring
As a complement to its REST API, the test bed also publishes a health-check endpoint to facilitate availability monitoring. This is not listed as a part of the other REST operations, nor does it figure in the OpenAPI documentation as it is always available regardless of whether the REST API is enabled or not.
To make a health-check, send a GET
to path /api/healthcheck
. If all services are up and running the response will have a code 200
(OK) and
empty body.
Besides testing as part of availability monitoring, this operation could also be used as part of automated build scripting to identify when the test bed has completed its initialisation and is ready to receive other API calls.